[Solved] Angular HTTP GET with TypeScript error http.get(…).map is not a function in [null]
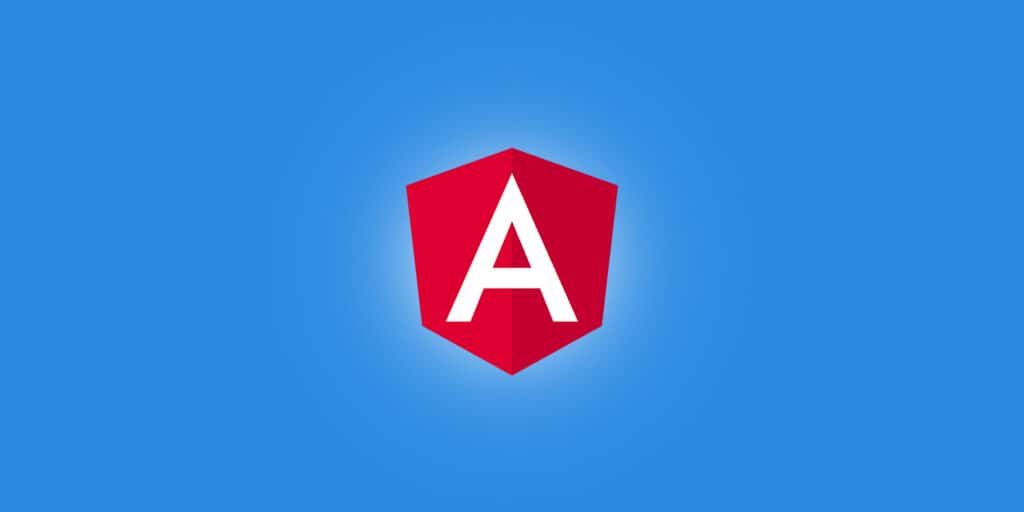
Problem:
I have a problem with HTTP in Angular.
Service classimport {Injectable} from "angular2/core"; import {Hall} from "./hall"; import {Http} from "angular2/http"; @Injectable() export class HallService { public http:Http; public static PATH:string = 'app/backend/' constructor(http:Http) { this.http=http; } getHalls() { return this.http.get(HallService.PATH + 'hall.json').map((res:Response) => res.json()); } }
HallListComponent
export class HallListComponent implements OnInit { public halls:Hall[]; public _selectedId:number; constructor(private _router:Router, private _routeParams:RouteParams, private _service:HallService) { this._selectedId = +_routeParams.get('id'); } ngOnInit() { this._service.getHalls().subscribe((halls:Hall[])=>{ this.halls=halls; }); } }
However, I got an exception:
TypeError: this.http.get(...).map is not a function in [null]Solution:
The RxJS library is quite large. Size matters when we build a production application and deploy it to mobile devices. We should include only those features that we actually need.
Accordingly, Angular exposes a stripped down version of Observable in the rxjs/Observable module, a version that lacks almost all operators including the ones we'd like to use here such as the map method.
It's up to us to add the operators we need. We could add each operator, one-by-one, until we had a custom Observable implementation tuned precisely to our requirements.
So as @Thierry already answered, we can just pull in the operators we need:
Import { map } 'rxjs/add/operator/map'; Import { delay } 'rxjs/operator/delay'; Import { mergeMap } 'rxjs/operator/mergeMap'; Import { switchMap } 'rxjs/operator/switchMap';
Pipeable operators are pure functions and do not patch the Observable. You can import operators using the ES6 import syntax
import { map } from "rxjs/operators"
and then wrap them into a function pipe() that takes a variable number of parameters, i.e. chainable operators.
Something like this -
getHalls() { return this.http.get(HallService.PATH + 'hall.json') .pipe( map((res: Response) => res.json()) ); }