[Solved] Handle response - SyntaxError: Unexpected end of input when using mode: 'no-cors'
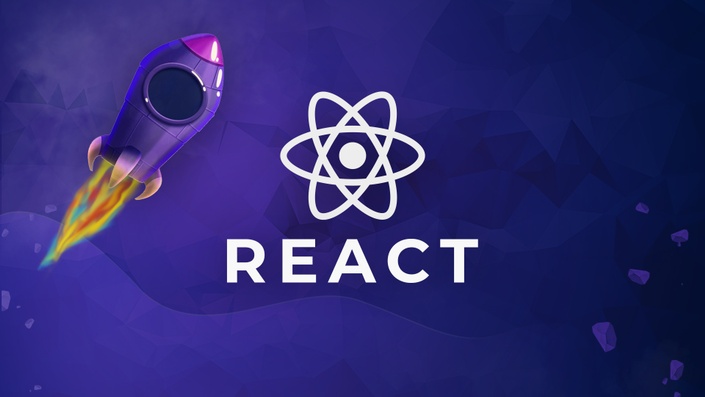
We call a REST-API and want to handle the response. The call works, we get a response, that we see in chrome dev tools.
Here is the code :
function getAllBooks() { fetch('http://localhost:8080/book', { method: 'POST', mode: 'no-cors', credentials: 'same-origin', headers: { 'Accept': 'application/json', 'Content-Type': 'application/json', }, body: JSON.stringify({ objectClass: 'course', crud: '2' }) }).then(function (response) { console.log(response); return response.json(); }).catch(function (err) { console.log(err) }); }
we get a "SyntaxError: Unexpected end of input" at line number 16
return response.json();
The console.log looks like this:
Solution:
You need to remove the mode: 'no-cors' setting from your request. Setting no-cors mode is exactly the cause of the problem you’re having. A no-cors request makes the response type opaque. The opaque means your frontend JavaScript code can’t see the response body or headers. https://developer.mozilla.org/en-US/docs/Web/API/Request/mode explains:
no-cors — JavaScript may not access any properties of the resulting Response
So the effect of setting mode: 'no-cors' is essentially to tell browsers, “Don’t let frontend JavaScript code access the response body or headers under any circumstances.” Imagine you’re setting mode: 'no-cors' for the request because the response from http://localhost:8080/book doesn’t include the Access-Control-Allow-Origin response header or because your browser’s making an OPTIONS request to http://localhost:8080 because your request is one that triggers a CORS preflight, and so your browser’s doing an OPTIONS preflight.