How do I conditionally add attributes to React components?
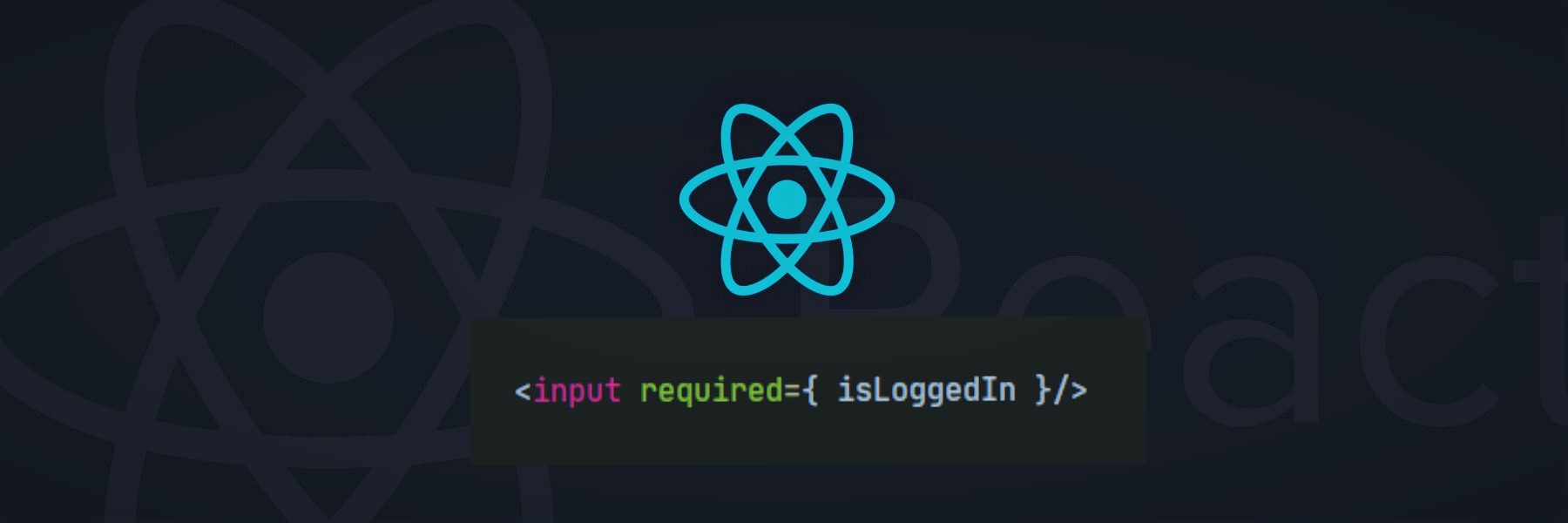
Rendering a React Component is easy. But sometimes such a situation comes up that, you only need to add attributes to a React Component if a certain condition is met. Or you need to send your Parent Component's state value to Child Component then add an attribute in your component if your desired condition is met.
In this article, we will implement the technique to add conditional rendering to your React Component.
Let's develop a simple app where we can add Disabled and Required attributes in our input field and change the attributes by clicking on a button. Also, we will show how to send the Parent Component's state value to Child Component and do the same thing.
First, you have to set your attribute status in your component state. Then, make a function for the onclick event of a button to change the status of the attribute. In the function, you have to update your new attribute status in the setState method. Finally, in the render method, you just have to call the state value in your tag attribute.
Here’s the example code:
class ParentComponent extends Component { state = { disabled: true, required: false, text: "Change to Required & Enabled" } updateState = () => { let text = !this.state.disabled ? "Change to Required & Enabled" : "Change to Not-Required & Disabled"; this.setState({ disabled: !this.state.disabled, required: !this.state.required, text: text }) } render() { return ( <div> <input disabled={this.state.disabled} required={this.state.required}/> <ChildComponent disabledAction={this.state.disabled} requiredAction={this.state.required} /> <button onClick={this.updateState}> {this.state.text} </button> </div> ); } }
Look, here we have used a component named ChildComponent. If you want to use your state value in your child component and do the same condition check, you can do it like this. Like the above example, you just need to send the parent state value as props to your child component.
Here is the example code of the ChildComponent,
class ChildComponent extends Component { render() { return ( <div> <input disabled={this.props.disabledAction} required={this.props.requiredAction}/> </div> ); } }
React is smart enough to NOT pass a prop value if the value is false.
And your feature is implemented successfully!