How do I test that a child component is rendered?
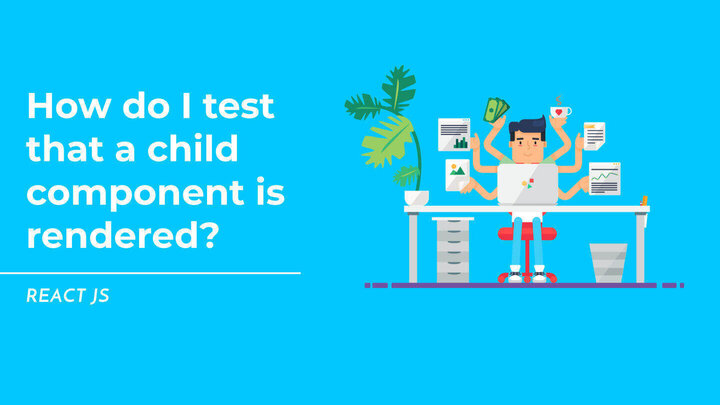
Problem:
You are creating a react-app and have many child component. Now, you are doing unit test. in this article I will talk about how do I test that a child component is rendered?
const ParentComponent = observer(({ props }) => {
const data = toJS(props.user);
return (
<div>
{data.map(user=> {
return <ChildComponent key={user.id} name={user.naem} />;
})}
</div>
);
});
this is how you do it:
Solution 1:
For this, you can use Enzyme for testing your react components. By using enzyme you can check if a parent component rendered its child component.
For this use containsMatchingElement().
Example:
describe('Parent Component', () => {
it('renders Child component', () => {
const wrapper = shallow(<ParenComponent props={props} />);
expect(wrapper.containsMatchingElement(<ChildComponent)).toEqual(true);
});
});
Solution 2:
You can also test the child component to be sure that it is rendered by simply referencing it by its displayName. So for that, you have to set displayName to your child Component. You can do that like this:
childComponent.displayName = “child-component”
Now you can test it like this:
const wrapper = shallow();
expect(wrapper.find('child-component’)).to.have.lengthOf(1);
Thank you very much for reading this article. If you have any type of testing problem or any questions you can comment below.