How do you check a checkbox in react-testing-library?
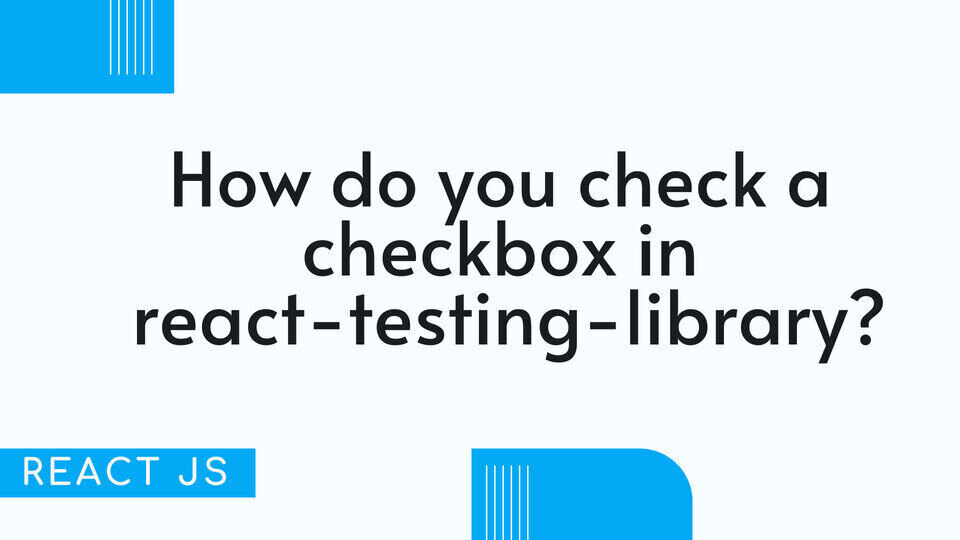
Problem:
Suppose you created a react app. Now you are trying to test it using react-testing-library.
You have a dropdown with display: none. If you click a checkbox that becomes displayed: block. The test is failing of the second expected block saying it should be displayed: none.
This is my code:
expect(getByLabelText('exampleCheckbox')).toHaveStyle(`
display: none;
`)
getByLabelText('exampleCheckbox').checked = true
expect(getByLabelText('exampleCheckbox')).toHaveStyle(`
display: block;
`)
In this article, we will learn how do you check a checkbox in the react-testing-library?
Solution:
You can test the checkbox using react-testing-library, You just have to fireEvent.click the checkbox.
In my code, there is a basic div with style display: none or display: block what is based on whether a checkbox is checked or not. By using react hooks it maintains the state.
In the react-testing-library, there is also a test that demonstrates this checking the checkbox and then asserting the changes style.
So, this is what the code looks like:
it('changes style of div as exampleCheckbox is checked or unchecked', () => {
const { getByTestId } = render(<ExampleCheckbox />)
const exampleCheckbox = getByTestId(CHECKBOX_ID)
const div = getByTestId(DIV_ID)
expect(exampleCheckbox.checked).toEqual(false)
expect(div.style['display']).toEqual('none')
fireEvent.click(exampleCheckbox)
expect(exampleCheckbox.checked).toEqual(true)
expect(div.style['display']).toEqual('block')
fireEvent.click(exampleCheckbox)
expect(exampleCheckbox.checked).toEqual(false)
expect(div.style['display']).toEqual('none')
})
Thanks for reading the post. If you have any question please comment below.