How to add type check for history object in React using TypeScript?
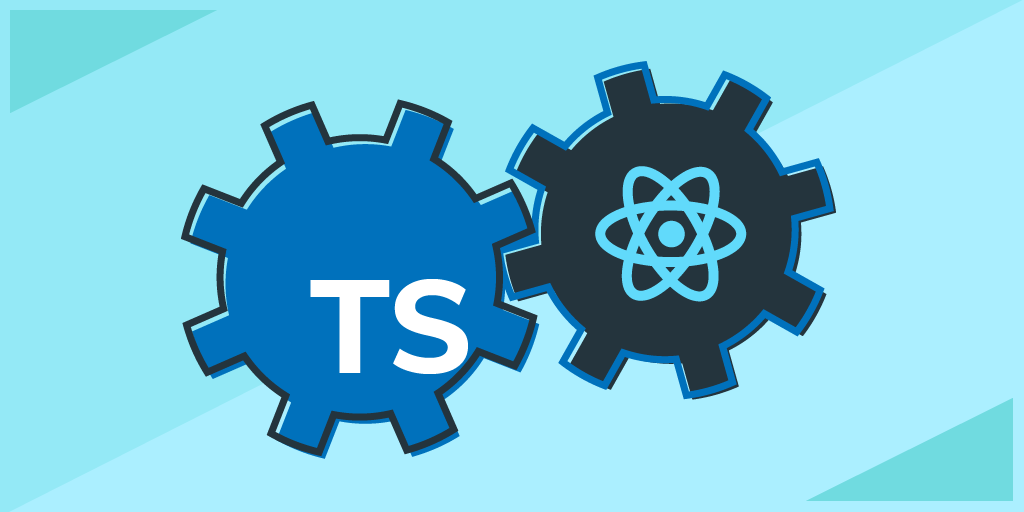
If we want to easily read and debug the React app, we must use typescript. it makes our code easier to understand. With TypeScript, we can make a huge improvement over plain JavaScript in our code. But sometimes we face some issues to define a type. History type checks in react one of them. In this article, I will try to explain how can we do it in our react app.
We can use the RouteComponentProps interface, which declares all props passed by withRouter:
import { RouteComponentProps } from 'react-router-dom';
..
interface ChildProps extends RouteComponentProps<any> {
/* other props for ChildComponent */
}
const ChildComponent : React.FC<ChildProps> = ({ history }) => (
..
);
Alternatively, if history doesn't come from withRouter but is passed by itself as a prop, we can import the type from history:
import { History } from 'history';
..
interface ChildProps {
history : History
/* other props for ChildComponent */
}
const ChildComponent : React.FC<ChildProps> = ({ history }) => (
..
);
Hopefully its can help to you to fix your problem. If you face another issue, you could feel free to contact with us.