How to check whether an array contains a string in TypeScript?
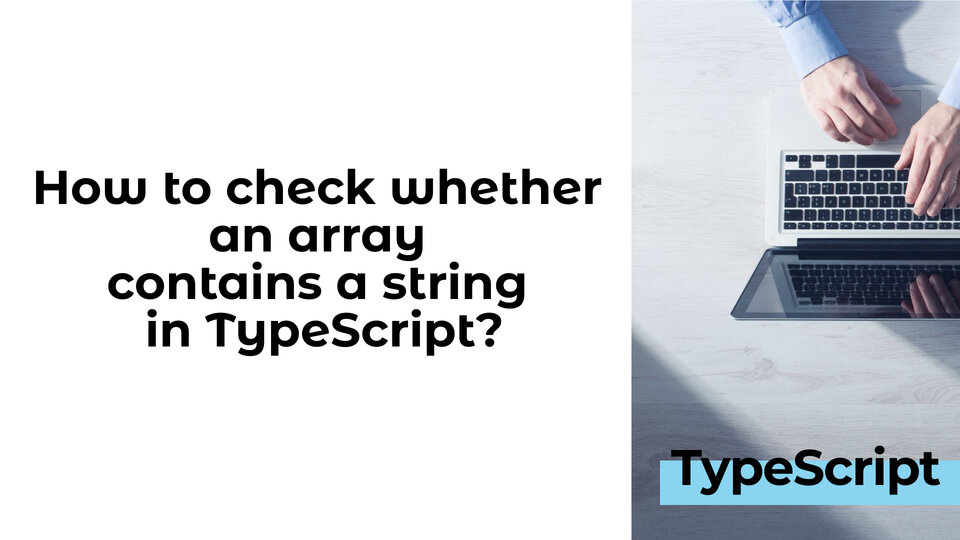
Problem:
I have an array in TypeScript. And now I want to check in my subjectArray contains the string "math".
const subjectArray: string[] = ["english", "math", "science"];
In this article, we are going to learn how to check whether an array contains a string in TypeScript?
Solution:
In the prototype of arrays, there are many utility methods for an array. There are multiple ways you can achieve this goal. So, here are a few which are convenient for this:
1. indexOf(): This method takes any value as an argument. If the value is in your array then it returns the index at which the element can be found in the array and if the value is not found in your array it will give you -1.
const indexOf = subjectArray.indexOf("math")
console.log(indexOf) // 1
2. includes(): This includes() method also takes any value as an argument. If the value presents it the method returns true. If not it will give you false.
console.log(subjectArray.includes("math");) // true
3. filter(): This filter method has a callback function. It will return an array. If the array contains the string we want it will return with that string.
console.log(subjectArray.filter(el=> el === "math")) // ["math"]
If not it will give us an empty array.
console.log(subjectArray.filter(el=> el === "engineering ")) // []
Thank you for reading this article. Hope this solves your problem. If you have any more questions you can comment below.