How to convert a string to enum in TypeScript?
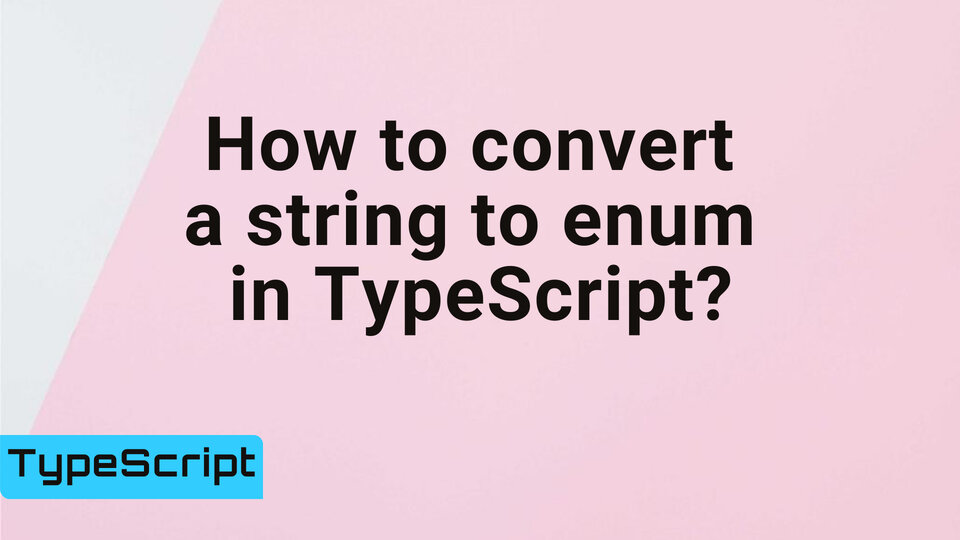
Problem:
I am new to TypeScript. I have defined the following enum Color in TypeSricpt.
enum Name{
Joe, Steve
}
But when I try the following code:
const steve= "Steve";
const name : Name = <Name>steve;
This gives me an error. So, how can I this value to an enum?
In this article, we are going to learn how to convert a string to enum in TypeScript?
Solution:
The simple solution is you can create a parse function on the enum which accepts one of the keys as arguments. When a new string is added no changes are needed.
enum Car { Tesla, Lamborghini }
Get the keys 'tesla' | 'lamborghini' (but not 'parse')
type CarKey = keyof Omit<typeof Car, 'parse'>;
namespace Car {
export function parse(carName: CarKey ) {
return Car[carName];
}
}
The key 'tesla' exists as an enum so no warning is given.
Car.parse('tesla'); // == Cars.tesla
Without the 'any' cast you would get a compile-time warning. Because 'xyz' is not one of the keys in the enum.
Car.parse('xyz' as any); // == undefined
Creates warning: "Argument of type '"bar"' is not assignable to parameter of type '"tesla" | "lamborghini"'.
Car.parse('bar');
Thank you for reading this article. If you have any more questions you can comment below.