How to dynamically assign properties to an object in TypeScript?
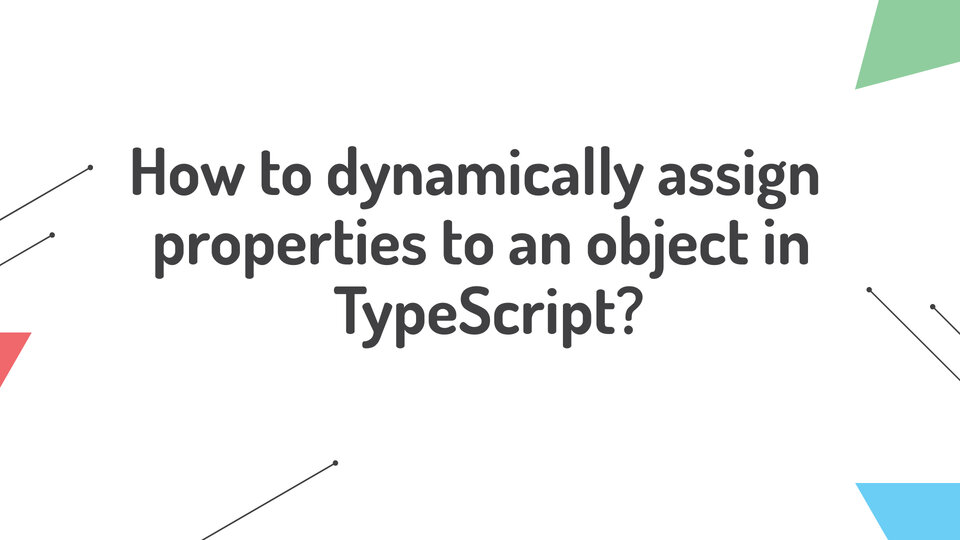
Problem:
Normally you can add a property to an object like this:
const myObj = {};
myObj.newProperty = "new value";
But in TypeScript, it is giving me an error. it says,
the "newProperty" does not exist on the value of type "{}"
So in this article, I am going to talk about how to dynamically assign properties to an object in TypeScript.
Solution:
myObj = {} this means myObj is Object. So if you make in any it defeated the purpose of using typescript.
const myObj = <any>{}
So to do it the right way we can define an interface like this:
interface ExampleObject {
typesafeProp1: number,
[key: string]: any
}
const myObj: ExampleObject = {};
or you can make it compact:
const myObj: {[k: string]: any} = {};
ExampleObject can now accept any string as key and any type as value:
myObj.newProperty = "new value";
myObj.newProperty2 = 999;
Update:
There is a new Utility type in typescript Record<Keys, Type>. For key-value pairs where property names are unknown, it is a much cleaner alternative.
Let's compare them:
This:
const myObj: {[k: string]: any} = {};
becomes:
const myObj: Record<string,any> = {}
so now ExampleObject can be defined by extending Record type:
interface ExampleObject extends Record<string,any> {
typesafeProp1?: number,
requiredProp1: string,
}
Hope this solves your problem. If you have any type of problem please comment below.