How to extend types in Typescript?
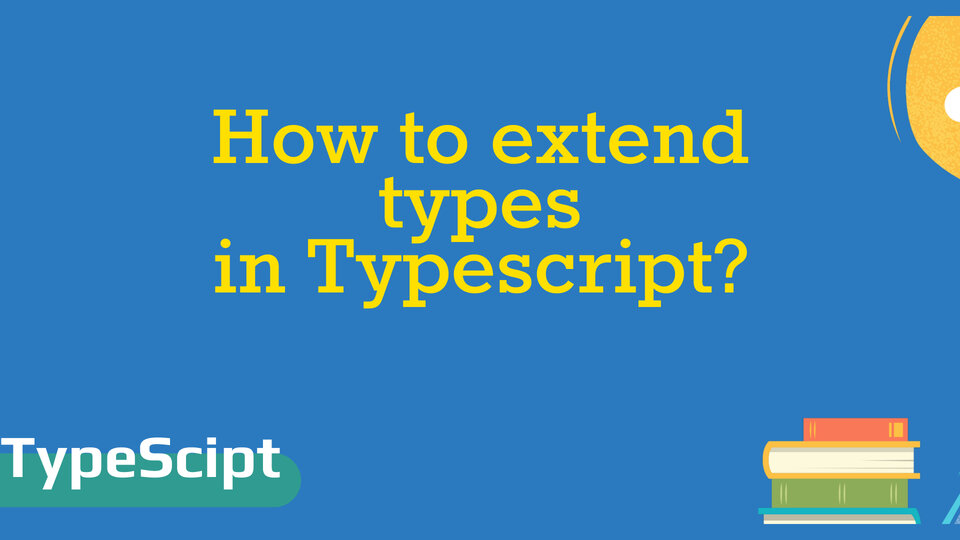
Problem:
I have created a type in my TypeScript project. Now I want to add more to this type.
type Book = {
name: string;
releaseDate: Date;
genre: string;
}
I want to extend the type like this:
type BookPrice extends Book = {
price: number;
}
But this is giving me an error.
In this article, we are going to learn how to extend types in Typescript?
Solution 1:
The first mistake you are doing is using extends with type. The extends keyword only works with interfaces and classes. First, you can define the type like this:
type Book = {
name: string;
releaseDate: Date;
genre: string;
}
Now you can extend the Book type using the interface.
interface BookPrice extends Book {
price: number;
}
It will not work the other way round BookPrice must be declared as interface if you want to use extends syntax.
Solution 2:
You can also extend your type without using extends keyword. To do this you can use intersection type:
type LastName = {
lastName: string;
};
type FirstName = {
firstName: string;
};
type FullName = LastName & FirstName;
Now, you can use this extended type anywhere you want in your code.
const authorName: FullName = {
lastName: 'Stephen',
firstName: 'King',
};
Thank you for reading the article. If you face any problems please comment below.