[Solved] How to fix missing dependency warning when using useEffect React Hook?
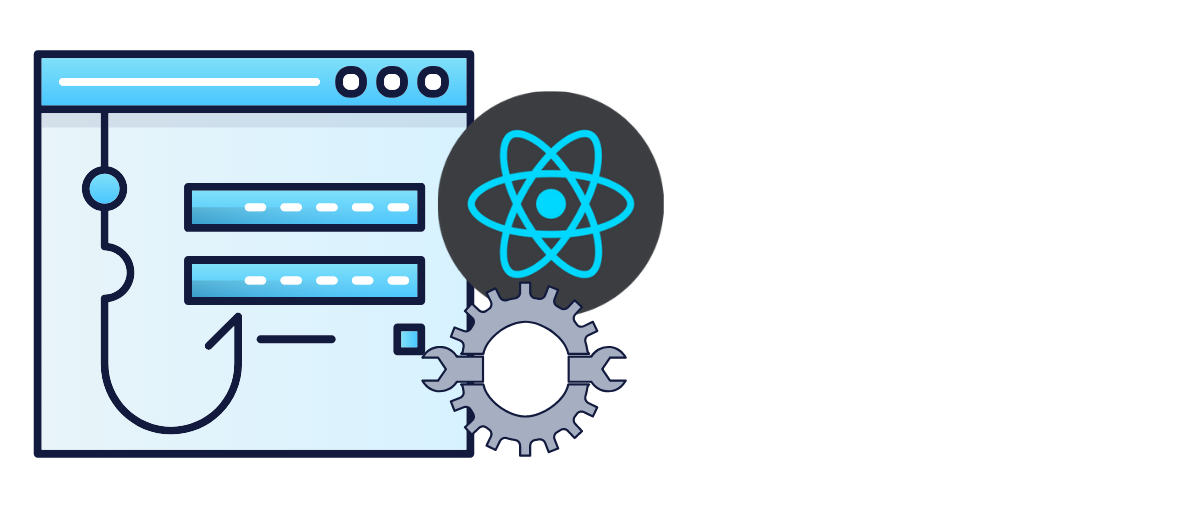
Problem:
Sometimes we get this error when we attempt to prevent an infinite loop on a fetch request:
./src/components/CarList.js Line 45: React Hook useEffect has a missing dependency: 'fetchCarList'. Either include it or remove the dependency array react-hooks/exhaustive-deps
Here is my code:
useEffect(() => { fetchCarList(); }, []);
const fetchCarList= () => { return fetch("theURL", {method: "GET"} ) .then(res => normalizeResponseErrors(res)) .then(res => { return res.json(); }) .then(rcvdCarList => { // some stuff }) .catch(err => { // some error handling }); };Solution:
Here we have included 3 solutions.
1. Declare function inside useEffect()
useEffect(() => { function fetchCarList() { ... } fetchCarList() }, [])2. Memoize with useCallback()
In this case, if you have dependencies in your function, you have to include them in the useCallback dependencies array. This will trigger the useEffect again if the function's params change. Besides, it has a lot of boilerplate, so you can just pass the function directly to useEffect.
const fetchCarList= useCallback(() => { ... }, []) useEffect(() => { fetchCarList() }, [fetchCarList])3. Disable eslint's warning
useEffect(() => { fetchCarList() }, []) // eslint-disable-line react-hooks/exhaustive-deps
Hope this solution will solve your problem. To learn more about react, you can check The complete React developer course w/Hooks & Redux course.