How to integrate the interceptor in angular?
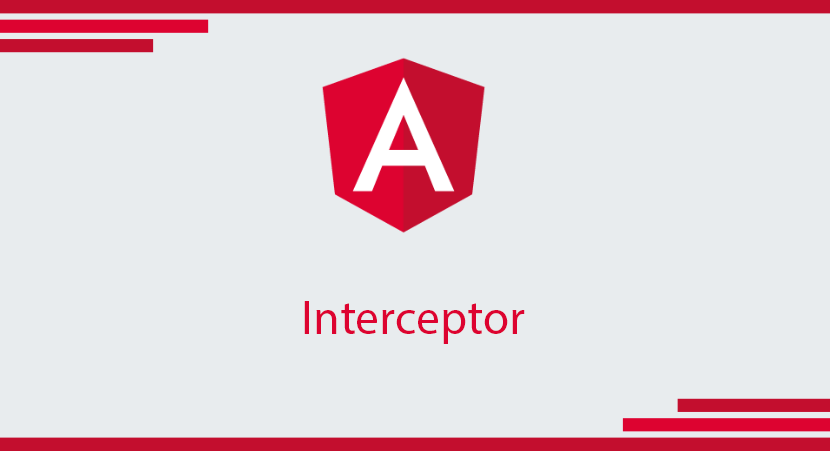
Interceptors provide a mechanism to intercept and/or mutate outgoing requests or incoming responses. They are very similar to the concept of middle-ware with a framework like Express, except for the front-end.
Why interceptors?
Interceptors can be really useful for features like caching and logging. Interceptors are a way to do some work for every single HTTP request or response.
- HTTP activity
- Add a token
- Catch HTTP responses
Basic Setup of interceptor
1st need to inject HttpInterceptor. Intercept method tages two arg req and next. Then return an observable HTTPEvent. req is the request object itself and is of type HTTP Request. Next is the HTTP handler, of type HTTP Handler. The handler has a handle method that returns our desired HttpEvent observable.
Create a service that implements HttpInterceptor:
import { HttpInterceptor} from '@angular/common/http'; import { Injectable } from '@angular/core'; @Injectable() export class TokenInterceptorService implements HttpInterceptor { intercept(req: HttpRequest<any>, next: HttpHandler): Observable<HttpEvent<any>> { // All HTTP requests are going to go through this method } }
It has to be added to the list of all HTTP_INTERCEPTORSM, which can be done that way in app.module.ts:
@NgModule({ ... providers: [{ provide: HTTP_INTERCEPTORS, useClass: TokenInterceptorService, multi: true }] })How we can use multiple interceptors?
You could define multiple interceptors with something like this
providers: [ { provide: HTTP_INTERCEPTORS, useClass: MyInterceptor, multi: true }, { provide: HTTP_INTERCEPTORS, useClass: MySecondInterceptor, multi: true }],