How to pass params with history.push/Link/Redirect in react-router v4?
Article
Tarif Hossain
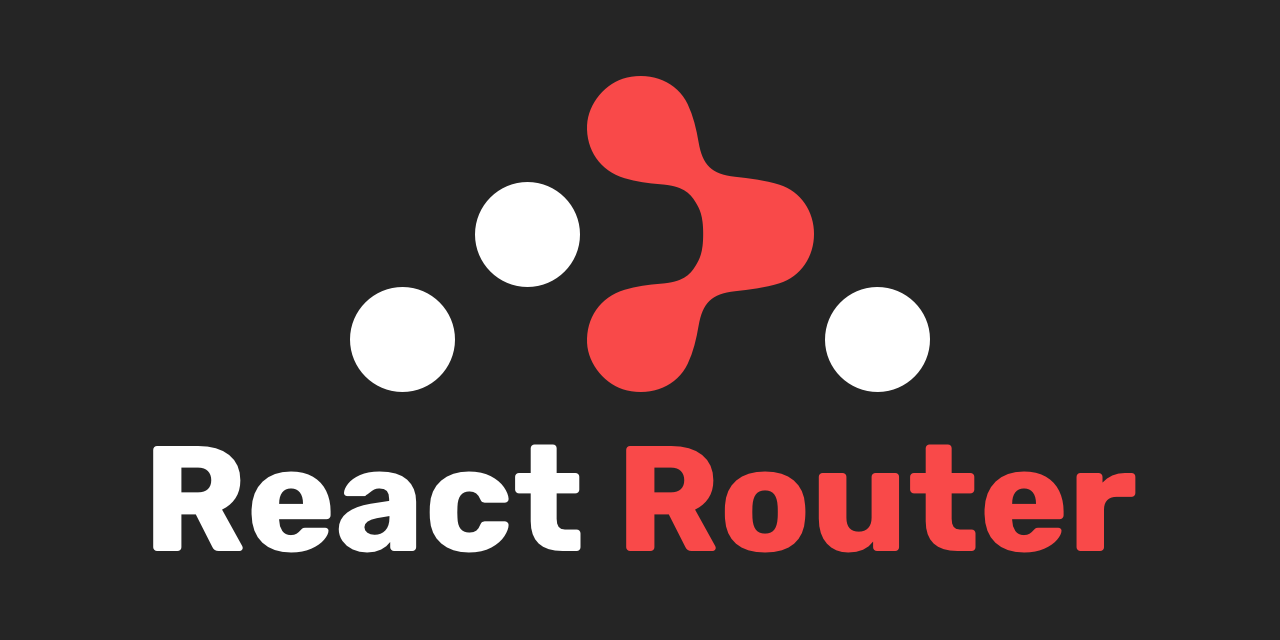
How can we pass parameter with this.props.history.push('/page') in React-Router v4?
.then(response => { var r = this; if (response.status >= 200 && response.status < 300) { r.props.history.push('/template'); });
Solution:
First of all, you need not do var r = this; as this in if statement refers to the context of the callback itself which since you are using arrow function refers to the React component context.
So while navigating you can pass props to the history object like
this.props.history.push({ pathname: '/template', search: '?query=abc', state: { detail: response.data } })
or similarly for the Link component or the Redirect component
<Link to={{ pathname: '/template', search: '?query=abc', state: { detail: response.data } }}> My Link </Link>
The solution for use with React hooks (16.8 onwards):
package.json (always worth updating to latest packages) { "react": "^16.12.0", "react-router-dom": "^5.1.2", }Passing parameters with history push:
import { useHistory } from "react-router-dom"; const FirstPage = props => { let history = useHistory(); const someEventHandler = event => { history.push({ pathname: '/secondpage', search: '?query=abc', state: { detail: 'some_value' } }); }; }; export default FirstPage