How to read text file in react
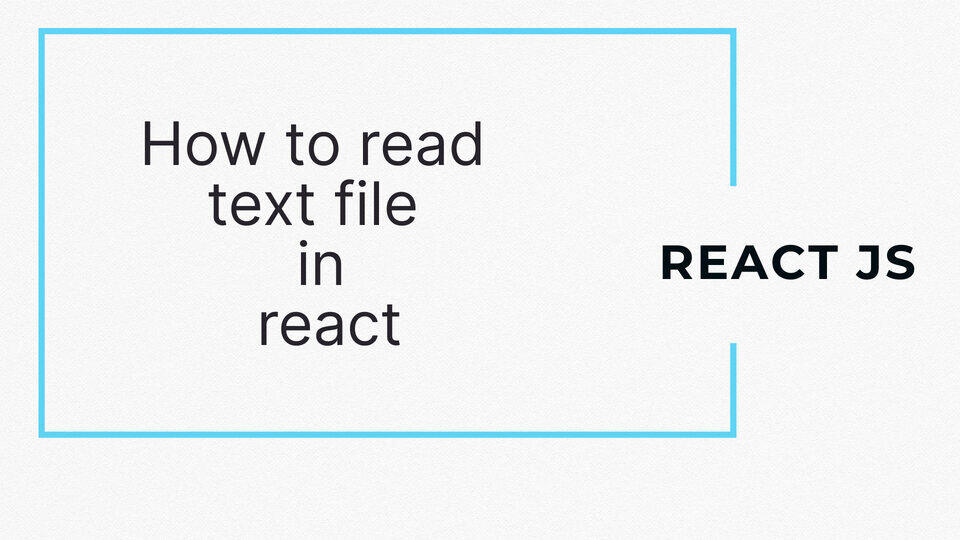
Problem:
You created a react js app. You want to read a text file from one page. But when you import the text file and execute the code you get HTML code in the console log. In this article, we are going to learn how to read text files in react.
import React form 'react';
import exampleTxt from './exampleTxt.txt'
class App extends React.Component {
clickHandler = () => {
fetch("exampleTxt.txt")
.then(function(res){
return res.text();
}).then(function (data) {
console.log(data);
})
}
render() {
return(
<div>
<button onClick={this.clickHandler}>Click Here</button>
</div>
)
}
}
Solution 1:
To get the .txt file you have to import the file first:
import raw from '../constants/foo.txt';
Now all you have to do is fetch it and transform it into text:
clickHandler = () => {
fetch(exampleTxt.txt)
.then(t => t.text()).then(text => {
console.log('you text', text)
})
}
Solution 2:
import React, { Component } from 'react';
class App extends Component {
constructor(props) {
super(props);
}
exampleFile = async (e) => {
e.preventDefault()
const exampleFileReader = new FileReader()
exampleFileReader.onload = async (e) => {
const text = (e.target.result)
console.log(text)
alert(text)
};
exampleFileReader.readAsText(e.target.files[0])
}
render = () => {
return (
<div>
<input type="file" onChange={(e) => this.exampleFile(e)} />
</div>
)
}
}
export default App;
You can also read your text using the input field as shown above.
Solution 3:
Let's say you created a file called data.js and in there you have your text. Like this:
const exampleText= "My Text goes here";
export default exampleText;
And now you want to read this text from another page. You can just import your text like this:
import { exampleText } from './data.js'; // Relative path to your file
console.log(exampleText);
I hope this article helps you with the problem you are having. If you have anymore question you can comment below.