How to redirect to the previous page in Django after a POST request
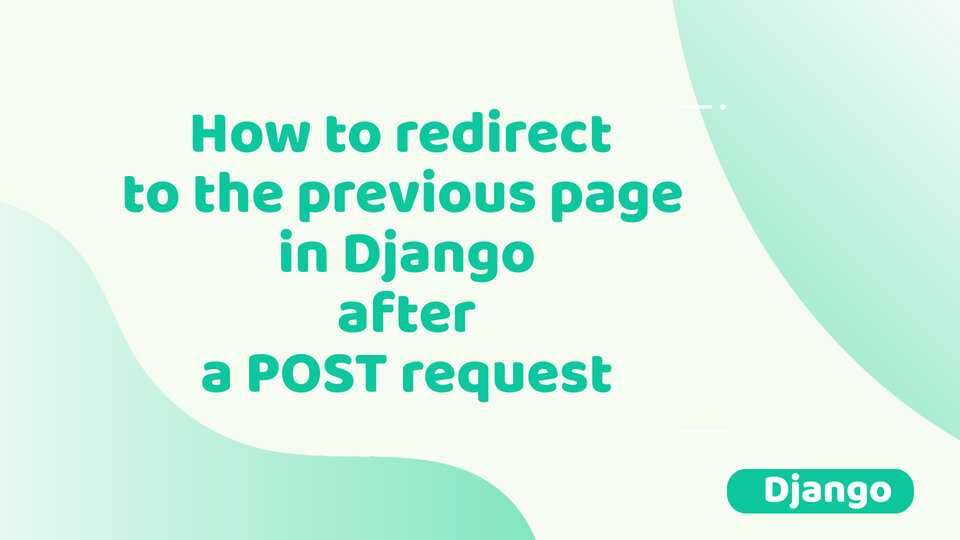
Problem:
I am creating a Django project. There is a navbar where you have a button. Using this button you can go to a page and create new content. I can redirect users to the main page. But, I want them to redirect back to the page where they came from. This is my code:
def createData(request):
uw = getuw(request.data.username)
if request.method =='POST':
form = AdverForm(request.POST, request.FILES)
if form.is_valid():
form.instance.data = request.data
form.save()
return HttpResponseRedirect('/', {'username': request.data.username, 'uw': uw})
args = {}
args.update(csrf(request))
args['username'] = request.data.username
args['form'] = AdverForm()
args['uw'] = uw
return render_to_response('createData.html', args)
So in this article, we are going to learn how to redirect to the previous page in Django after a POST request.
Solution 1:
You can define a field called next_page in you form and set this to request.path. Using this field you can redirect to the value of this path.
example.html
<form method="POST">
{% csrf_token %}
{{ form }}
<input type="hidden" name="next_page" value="{{ request.path }}">
<button type="submit">Submit</button>
</form>
view.py
next_page = request.POST.get('next_page', '/')
return HttpResponseRedirect(next_page)
This is how the django.contrib.auth works. You pass through an intermediate page, By using a query string, you can pass the "next_page" value.
link_page.html
<a href="{% url 'your_form_view' %}?next_page={{ request.path|urlencode }}">Let's Go To Form</a>
template.html
<form method="POST">
{% csrf_token %}
{{ form }}
<input type="hidden" name="next_page" value="{{ request.GET.next_page }}">
<button type="submit">Submit</button>
</form>
Solution 2:
You can also use the HTTP_REFERER value. But this will not work if use disabled sending referrer information. That means if the user uses private or incognito mode in the browser.
return HttpResponseRedirect(request.META.get('HTTP_REFERER', '/'))
Hope this solves the problem you are having. If you have any more questions please comment below