How to use onload in react?
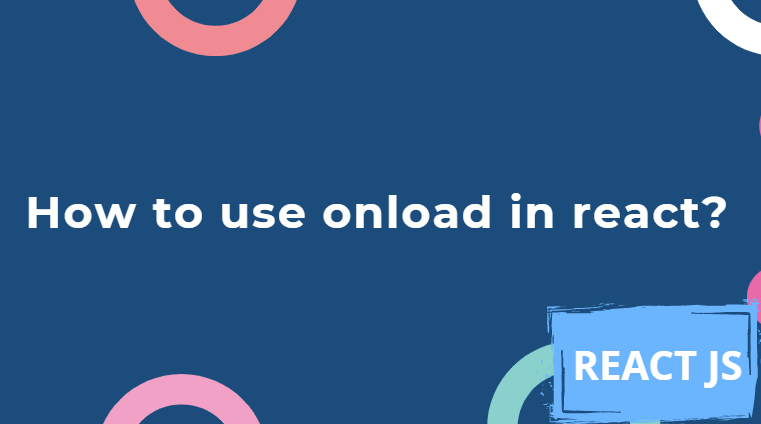
Problem:
I am now working on a project using react. I am trying to execute a function onload but seems it is not working. So, in this article, I am going to write about how to use onload in react.
My code is this:
class AvatarList extends React.Component {
constructor(props) {
super(props);
this.state = {
avatarArray: []
};
this.avatarStore = this.avatarStore.bind(this);
}
render() {
return (
<div>
<div onLoad= {() => this.avatarStore()}>
<img src="example.jpg" className="hide" alt="example.jpg"/>
</div>
<Gallery url={this.state.avatarArray}/>
</div>
);
}
avatarStore()
{
const avatarUrl=this.props.apiKeys;
const objArray = Object.values(avatarUrl);
this.setState({
avatarArray: objArray
});
}
}
But I want something like this:
class AvatarList extends React.Component {
constructor(props) {
super(props);
this.state = {
avatarArray: []
};
this.avatarStore = this.avatarStore.bind(this);
}
render() {
return (
<div>
<div onLoad= {() => this.avatarStore()}>
</div>
<Gallery url={this.state.avatarArray}/>
</div>
);
}
avatarStore()
{
const avatarUrl=this.props.apiKeys;
const objArray = Object.values(avatarUrl);
this.setState({
avatarArray: objArray
});
}
}
Solution:
You could simply load it in componentDidMount. You do not have to render an image that you do not want. You can just call imageStore when the image is loaded.
class AvatarList extends React.Component {
constructor(props) {
super(props);
this.state = {
avatarArray: []
};
this.avatarStore = this.avatarStore.bind(this);
}
componentDidMount() {
const img = new Image();
img.onload =() => {
// image has been loaded
this.avatarStore()
};
img.src = 'example.jpg';
}
render() {
return (
<div>
<Gallery url={this.state.avatarArray}/>
</div>
);
}
avatarStore() {
const avatarUrl=this.props.apiKeys;
const objArray = Object.values(avatarUrl);
this.setState({
avatarArray: objArray
});
}
}
But if you intended to call imageStore when the component has fully loaded, in this case, you can just trigger this this.imageStore() in componentDidMount
class AvatarList extends React.Component {
constructor(props) {
super(props);
this.state = {
avatarArray: []
};
this.avatarStore = this.avatarStore.bind(this);
}
componentDidMount() {
this.avatarStore()
}
render() {
return (
<div>
<Gallery url={this.state.avatarArray}/>
</div>
);
}
avatarStore() {
const avatarUrl=this.props.apiKeys;
const objArray = Object.values(avatarUrl);
this.setState({
avatarArray: objArray
});
}
}
I hope this helps you. If you are still having problems please comment below.