[Solved] How to use Router.push with state in Nextjs
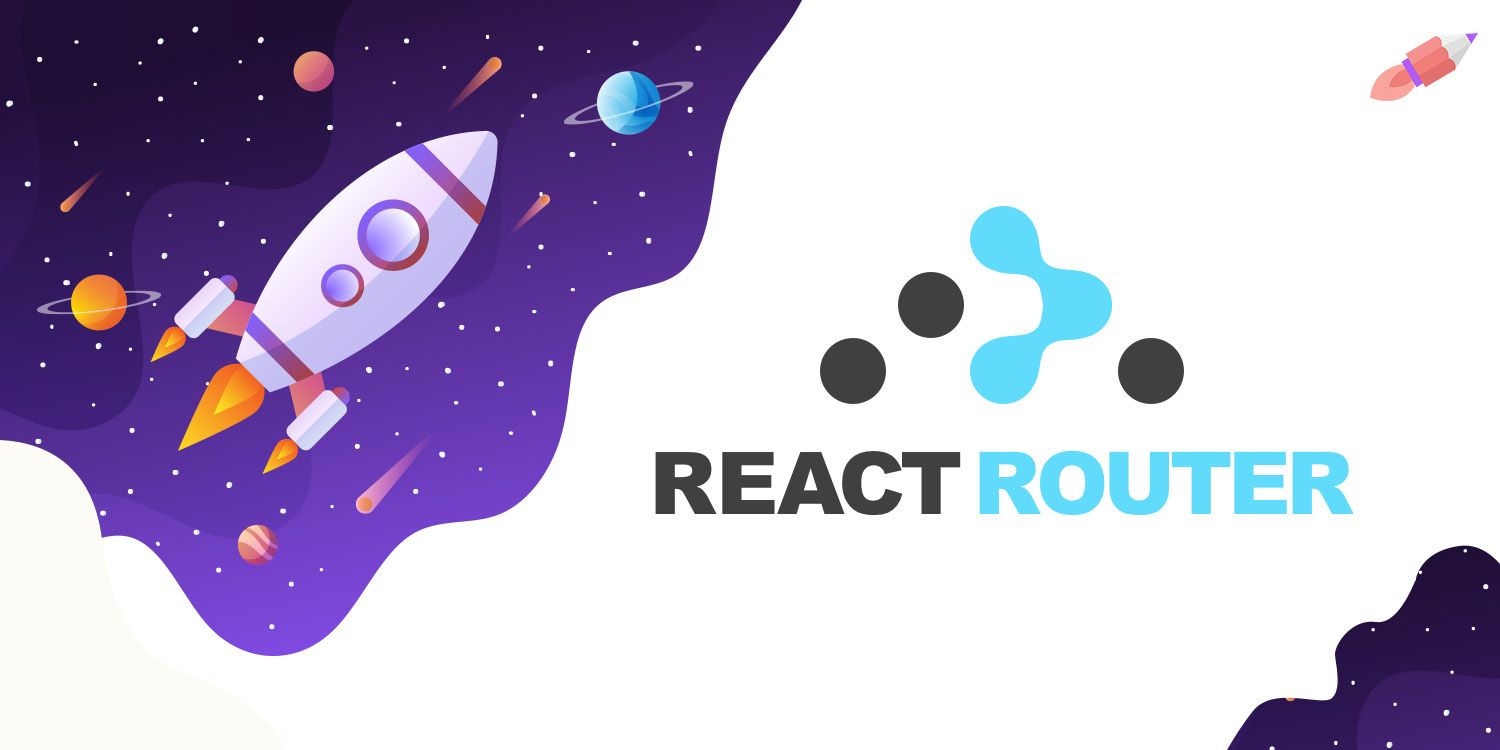
Problem:
I'm using next.js for rebuilding an app for server side rendering. I have a button that handles a search request.
In the old app, the handler was this one:
search = (event) => { event.preventDefault(); history.push({ pathname: '/results', state: { pattern: this.state.searchText, } }); }
In the results class, I could get the state date with this.props.location.state.pattern .
So now I'm using next.js:
performSearch = (event) => { event.preventDefault(); Router.push({ pathname: '/results', state: { pattern: this.state.searchText } }); };
Solution:
In next.js you can pass query parameters like this
Router.push({ pathname: '/about', query: { name: 'Someone' } })
pathname: String - Current route. That is the path of the page in /pages, the configured basePath or locale is not included.
query: Object - The query string parsed to an object. It will be an empty object during pre-rendering if the page doesn't have data fetching requirements. Defaults to {}
And then in your next page (here in /about page), retrieve the query via the router props, which needs to be injected to Component by using withRouter.
import { withRouter } from 'next/router'; class About extends Component { // your Component implementation // retrieve them like this // this.props.router.query.name } export default withRouter(About);
If you'll follow this steps, hope it works perfectly.