How to use 'theme' and 'props' in makeStyles?
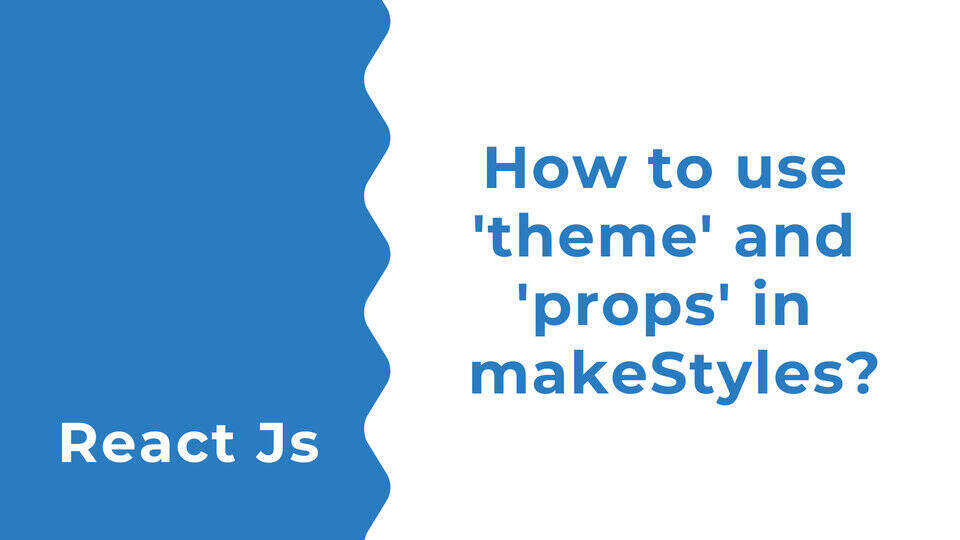
Problem:
You want to use both theme variables and props in your reactjs app. and you have tried this:
const customStyles = makeStyles(theme => ({
navBar: props => ({
background: "crimson",
borderBottom: theme.spacing(2),
}),
}));
const classes = useStyles(customColor);
customColor is a prop on the component with CSSProperties["customColor"]
But I am getting errors.
So, this is how to use 'theme' and 'props' in makeStyles.
Solution 01:
This solution is tested with:
"@material-ui/core": "^4.0.0-beta.1", "@material-ui/styles": "^4.0.0-rc.0", "@material-ui/core": "^4.12.X"
example-component.js
import React from 'react'; import { makeStyles } from '@material-ui/styles'; import { customStyles } from './example-component.styles.js'; const exampleComponent = (props) => { const styleProps = { background: "crimson", borderBottom: "2px"}; const classes = customStyles(styleProps); return ( <div className={classes.navBar}></div> // the given style will be applied ) }
example-component.styles.js
export const customStyles = makeStyles(theme => ({ navBar: props => ({ display: 'block', background: props.background, borderBottom: props.borderBottom, }), }));
TypeScript example:
example-component.ts
import React, { FC } from 'react'; import { makeStyles } from '@material-ui/styles'; import { customStyles } from './example-component.styles.ts'; import { exampleComponentProps, StylesProps } from './example-component.interfaces.ts'; const exampleComponent: FC<exampleComponentProps> = (props) => { const customStyleProps: StylesProps = { background: "crimson", borderBottom: "2px" }; const classes = useStyles(customStyleProps); return ( <div className={classes.navBar}></div> // the given style will be applied ) }
example-component.interfaces.ts
export interface CustomStyleProps { background: string; borderBottom: string; } export interface ExampleComponentProps { }
example-component.styles.ts
import { Theme } from '@material-ui/core'; import { makeStyles } from '@material-ui/styles'; import { CustomStyleProps } from './example-components.interfaces.ts'; export const customStyles = makeStyles<Theme, CustomStyleProps>((theme: Theme) => ({ navBar: props => ({ display: 'block', background: props.background, borderBottom: props.borderBottom, }), }));
Solution 02:
So the problem is you are passing an object to customStyles but you need to pass an object to customStyles.
So instead of doing this:
const classes = customStyles(customColor);
you should have:
const classes = customStyles(props);
or
const classes = useStyles({customColor});
Then you can get at customColor using:
color: theme.palette.getContrastText(props.customColor).
Here's a working example on codesandbox: check codesandbox here
Thank you for reading the article. I hope this solves the problem that you are having. If not please, comment below.