How to use useStyle to style Class Component in Material Ui
React JS
Faysal Shuvo
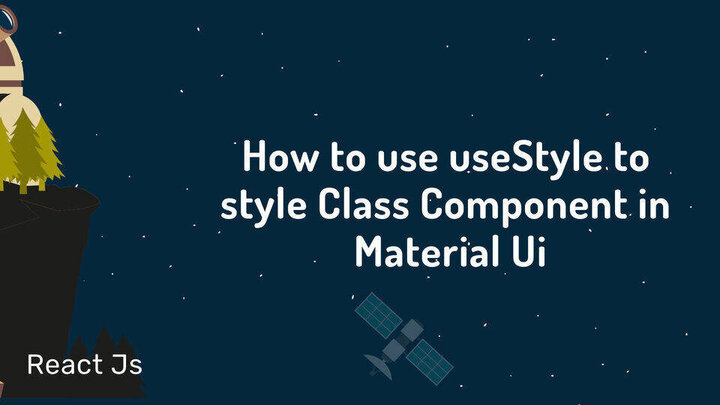
Problem:
You are using ReactJs. For styling, you want to use useStyle to style the Class Component. In this article, you are going to learn how to use useStyle to style Class Component in Material Ui.
import React,{Component} from 'react';
import Avatar from '@material-ui/core/Avatar';
import { makeStyles } from '@material-ui/core/styles';
import HomeIcon from '@mui/icons-material/Home';
const useStyles = makeStyles(theme => ({
'@global': {
body: {
backgroundColor: theme.palette.common.crimson,
},
},
paper: {
borderTop: theme.spacing(2),
display: 'flex',
},
img: {
border: theme.spacing(1),
backgroundColor: theme.palette.secondary.main,
}
})
);
class App extends Component{
const classes = useStyle();
render(){
return(
<div className={classes.paper}>
<Avatar className={classes.img}>
<HomeIcon />
</Avatar>
</div>
}
}
export default App;
But this styling is not working.
Solution 1:
You can style your component like this:
import { makeStyles } from '@material-ui/core/styles';
import LockOutlinedIcon from '@material-ui/icons/LockOutlined';
const useStyles = makeStyles(theme => ({
'@global': {
body: {
backgroundColor: theme.palette.common.white,
},
},
paper: {
marginTop: theme.spacing(8),
display: 'flex',
flexDirection: 'column',
alignItems: 'center',
},
avatar: {
margin: theme.spacing(1),
backgroundColor: theme.palette.secondary.main,
}
}));
class App extends Component {
state = {
searchNodes: ""
};
render() {
const { classes } = this.props;
return (
<div className={classes.paper}>
<Avatar className={classes.avatar}>
<LockOutlinedIcon />
</Avatar>
</div>
);
}
}
export default withStyles(styles)(App);
Solution 2:
You can replace makeStyles with withStyles and when you do const classes = useStyle(); replace this with const classes = useStyle
useStyle is not supposed to be a function. It is supposed to be a variable assignment.
Change this and your problem will be solved.
Solution 3:
useStyles is a react hook. React hook can only be used inside of the functional component. This is how you do it:
First, create a hook:
const useStyles = makeStyles(theme => {/* put your style here*/})
Now, you use it inside a functional component to create classes object:
const class=useStyles();
Then in JSX, you use the classes:
<div className={clases.avatar}
Thank you for reading this article. Hope this solves your problem. If not please comment down below.