[Solved] Module not found: Can't resolve 'firebase' in React js
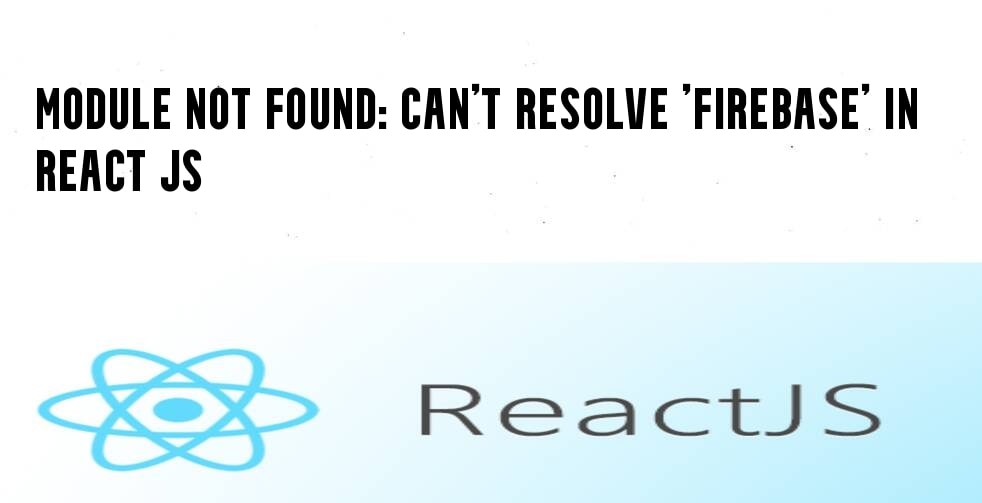
Problem:
While working on a React js project I was trying to import firebase into my application. I used npm i firebase command. Then I'm importing Firebase directly from Firebase, not from a file. So it showed an error like,
import firebase from 'firebase'; >in firebase.js file<
error in the terminal was,
./src/firebase.js Module not found: Can't resolve 'firebase' in 'C:\Users\Home\Documents\dsn\e\Documents..........'
Solution 1:
import { initializeApp } from 'firebase/app';
const firebaseConfig = {
//...
};
const app = initializeApp(firebaseConfig);
If you want to use older syntax then change your imports to compat libraries:
import firebase from "firebase/compat/app"
import "firebase/compat/auth"
import "firebase/compat/firestore"
You can read more about it in the documentation
Solution 2:
There should be no reason to downgrade to version 8 as version 9 provides a fully backward compatible import if you prefix the module import path with "compat". If you want to use older syntax then change your imports to compat libraries.
To use the older syntax:
import firebase from "firebase/compat/app";
// Other libraries might need to also be prefixed with "compat":
import "firebase/compat/auth";
import "firebase/compat/firestore
// Then you can then use the old interface, with version 9:
if (!firebase.apps.length) {
firebase.initializeApp(clientCredentials);
}
Thank you for reading the article. If you have any suggestions please comment below.