Multiple re-render on state change in react useState hook.
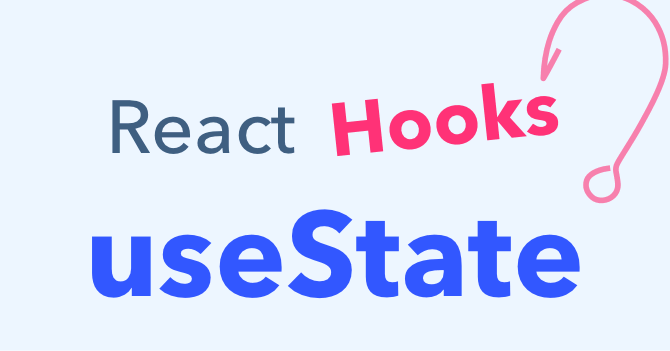
When we develop our React application we sometimes encounter that our components are being rendered more than once on state change. If we want to understand this issue, at first we need to understand how react works.
App componentimport { useState } from "react";
export default function App() {
const [count, setCount] = useState(1);
setInterval(() => {
setCount(count + 1);
}, 5000);
return (
<div className="App">
<p>{count}</p>
</div>
);
}
In App component after every 5 second count value is updated. So what happening in this case, after every 5 second "P" tag also re-render. Cause "P" tag represented "count" value. So how much times "P" tag re-render depend on how much times state is changed.
But sometimes we want to display the last result and we want to render our component just one times. So now I will try to explain it by another example.
Example componentimport {useEffect, useState} from "react";
export default function Example() {
const [count, setCount] = useState(1);
const [loading, setLoading] = useState(true);
useEffect(() => {
setTimeout(() => {
setCount(2);
setLoading(false)
}, 5000);
}, []);
if (loading) {
return <p>Loading ...</p>;
}
return (
<div className="App">
<p>{count}</p>
</div>
);
}
In Example component after 5 second count value will updated. But we want to render "P" tag once times which represented "count" value. So we used loading state to fix this issue. Initially we set loading value is "true". Set loading value is "false" when we got our final result. So in this case "P" tag is rendered once times which represented "count" value.