[Solved] ReflectionParameter::getClass() method deprecated in php 8.0.1
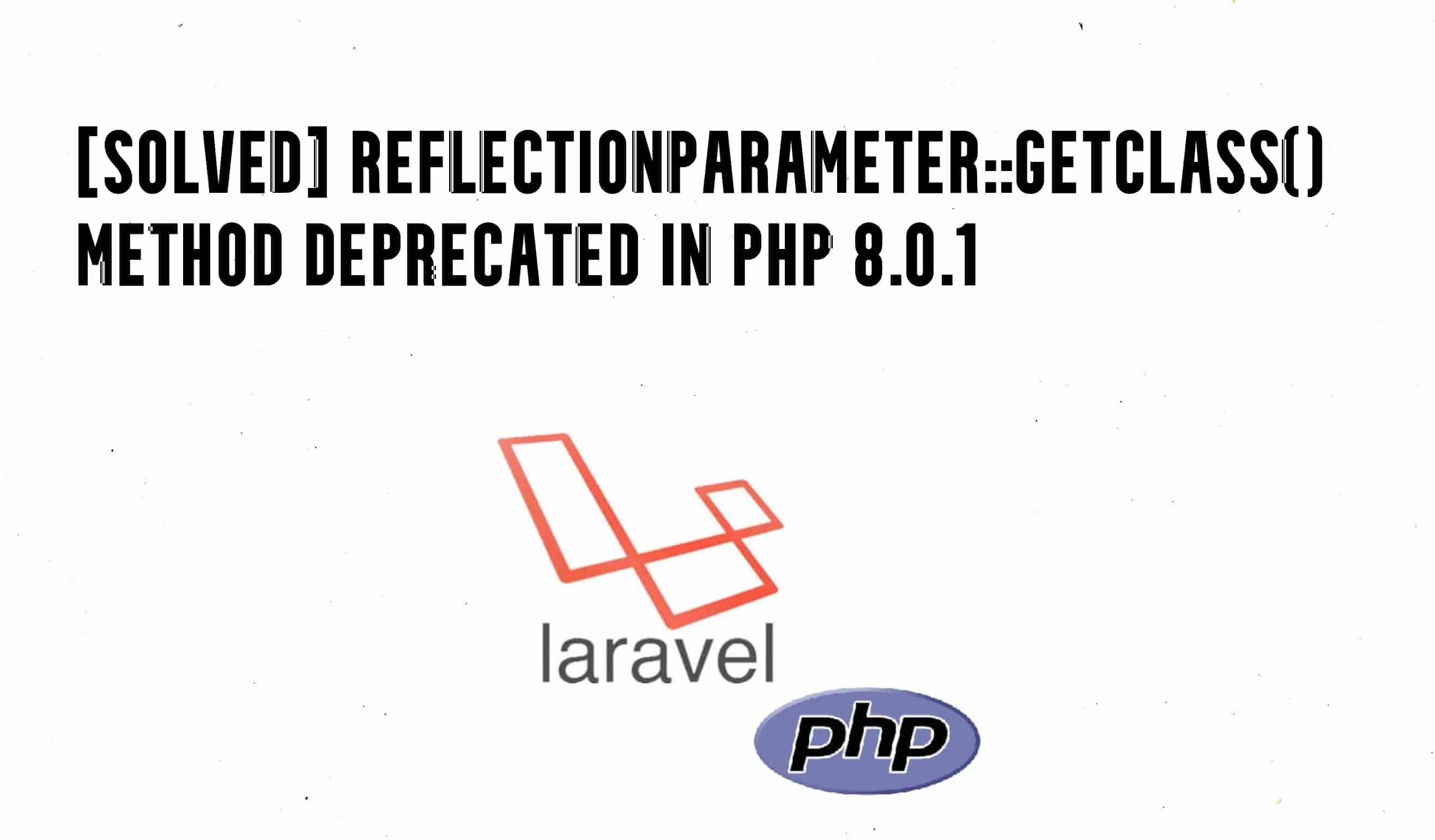
Problem:
If you are using PHP version 8 or above you may face an error that says,
PHP Fatal error: Uncaught ErrorException: Method ReflectionParameter::getClass() is deprecated
This error occurs because ReflectionParameter::getClass is no longer exists in PHP 8 or above. Several improvements to PHP type systems are included in PHP 8, including the addition of Union Types, mixed type, and a few more. In PHP 8, the following methods from ReflectionParameter class are deprecated:
ReflectionParameter::getClass() ReflectionParameter::isArray() ReflectionParameter::isCallable()
In this article, we will show you some quick solutions to the error.
Solution 1:
You can replace it with getType(), which the documentation suggests. You have to create your own ReflectionClass, from the ReflectionType.
$reflectionClass = new ReflectionClass($reflectionParam->getType()->getName());
If you want to make a ReflectionClass you can follow the code below.
class MyClass { public function test() { } } function someFunction(MyClass $param2) {} $reflectionFunc = new ReflectionFunction('someFunction'); $reflectionParams = $reflectionFunc->getParameters(); $reflectionType = new ReflectionClass($reflectionParams[0]->getType()->getName()); var_dump( $reflectionType); // MyClass
Solution 2:
If you are using laravel, the latest version of laravel 6, 7, and 8 have made changes required for PHP 8. All you have to do is complete the following steps:
- add PHP 8 to your composer.json (I've kept v7.4 just in case the production server does not support PHP 8 yet
"php": "^7.4|^8.0",
- run composer update to update your laravel to the latest version
composer update
- make sure to update the following libraries since they exist in all laravel applications
PHP to php:^8.0 Faker to fakerphp/faker:^1.9.1 PHPUnit to phpunit/phpunit:^9.3
- check for any other library which needs to be updated, contribute if they haven't supported PHP 8. but you should be good to go with most of the libraries since they have active contributors.
Thank you for reading the article. Hope the solutions will help you to solve your problem.