[Solved] TypeError: Cannot read properties of undefined (reading 'map') - React JS
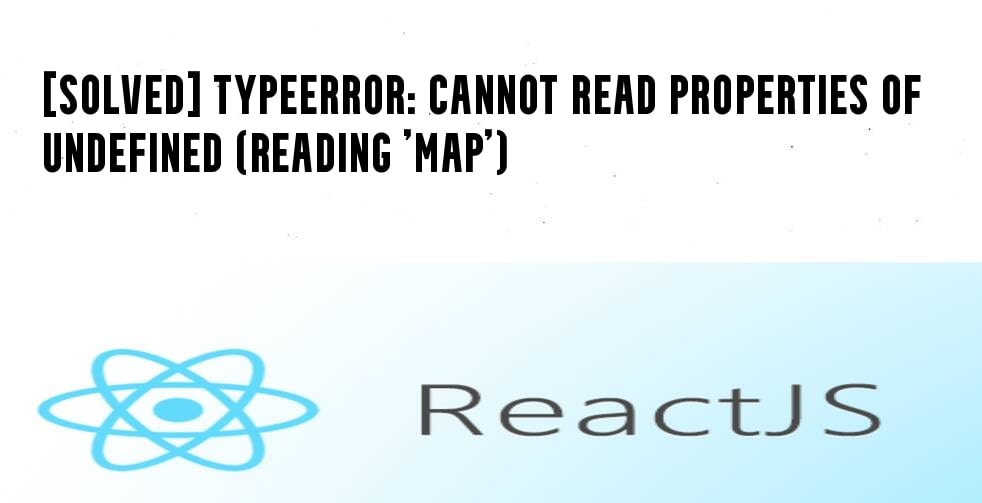
Problem:
Recently, we start developing a new web application project by React JS. But when we try to show the products list, we got this error. We will try to describe why this error occurs and how to fix it. The code is given below,
const products = [ {id: 1, name: 'Shoes', description: 'Running Shoes.' }, {id: 2, name: 'MacBook', description: 'Apple MacBook.' }, ]; const Products = ({ products }) => { const classes = useStyles(); return ( <main className={classes.content}> <div className={classes.toolbar} /> <Grid container justify="center" spacing={4}> {products.map((product) => ( <Grid item key={product.id} item xs={12} sm={6} md={4} lg={3}> <Product/> </Grid> ))}; </Grid> </main> ); };
The code has thrown an error saying,
TypeError: Cannot read properties of undefined (reading 'map')
Solution:
The problem arises because we're attempting to map an undefined object (products) into a JSX element. In our case which is the <Product />
Change this:
{products.map((products) => ( <Grid item key={product.id} item xs={12} sm={6} md={4} lg={3}> <Product/>
To this:
{products.map((products) => ( <Grid item key={products.id} item xs={12} sm={6} md={4} lg={3}> <Product/>
Thank you for reading the article. If you have any query please comment below.