[Solved] TypeError: Cannot read properties of undefined (reading 'map') ReactJs Getting data from a file
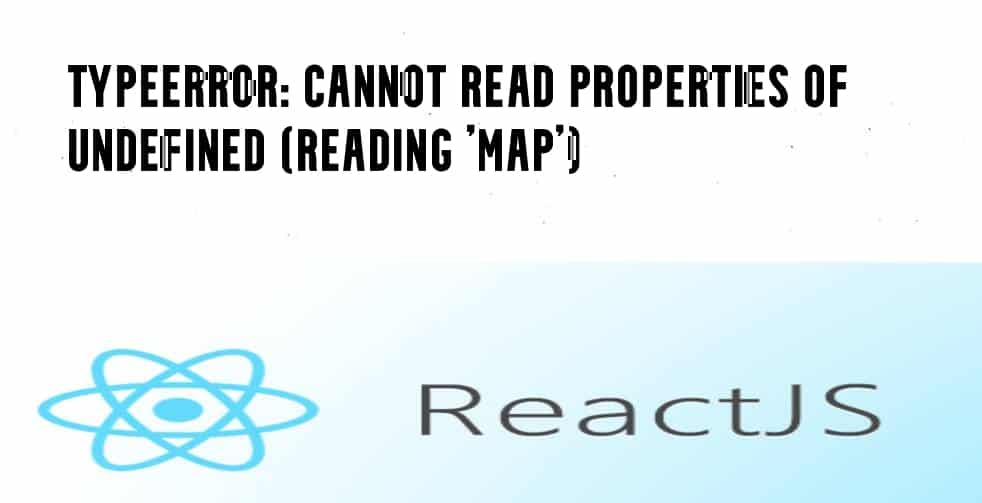
Problem:
While working with React Js, when we tried to show the list of posts using map, we got an error saying,
TypeError: Cannot read properties of undefined (reading 'map')
Our post object is in the following structure.
export const Posts = [ { id: 1, desc: "Love For All, Hatred For None.", photo: "assets/post/1.jpeg", date: "5 mins ago", userId: 1, like: 32, comment: 9, }, { id: 2, photo: "assets/post/2.jpeg", date: "15 mins ago", userId: 2, like: 2, comment: 1, },
Now we are mapping the data from that file.
export default function Feed() { return ( <div className="feed"> {Posts.map((p) => ( <Post key={p.id} post={p}/> ))} </div> ) }
Solution 1:
The error occurred because the Posts array is not defined. So when you are using the map function on Posts it is throwing the error.
So first you have to check if the Posts variable exists. You can do something like:
return (
<div className="feed">
<div className="feedWrapper">
<Share />
{(Posts || []).map(p => (
<Post key={p.id} post={p} />
))}
</div>
</div>
);
Solution 2:
You can solve the error by checking the length of Posts before looping through
export default function Feed() {
return (
<div className="feed">
<div className="feedWrapper">
<Share/>
{ Posts && Posts.length ?
Posts.map((p) => (
<Post key={p.id} post={p}/>
)) : null}
</div>
</div>
)
}
Thank you for reading the article. If you have any suggestions you can comment below.