[Solved] Uncaught TypeError: this.props.data.map is not a function - React JS
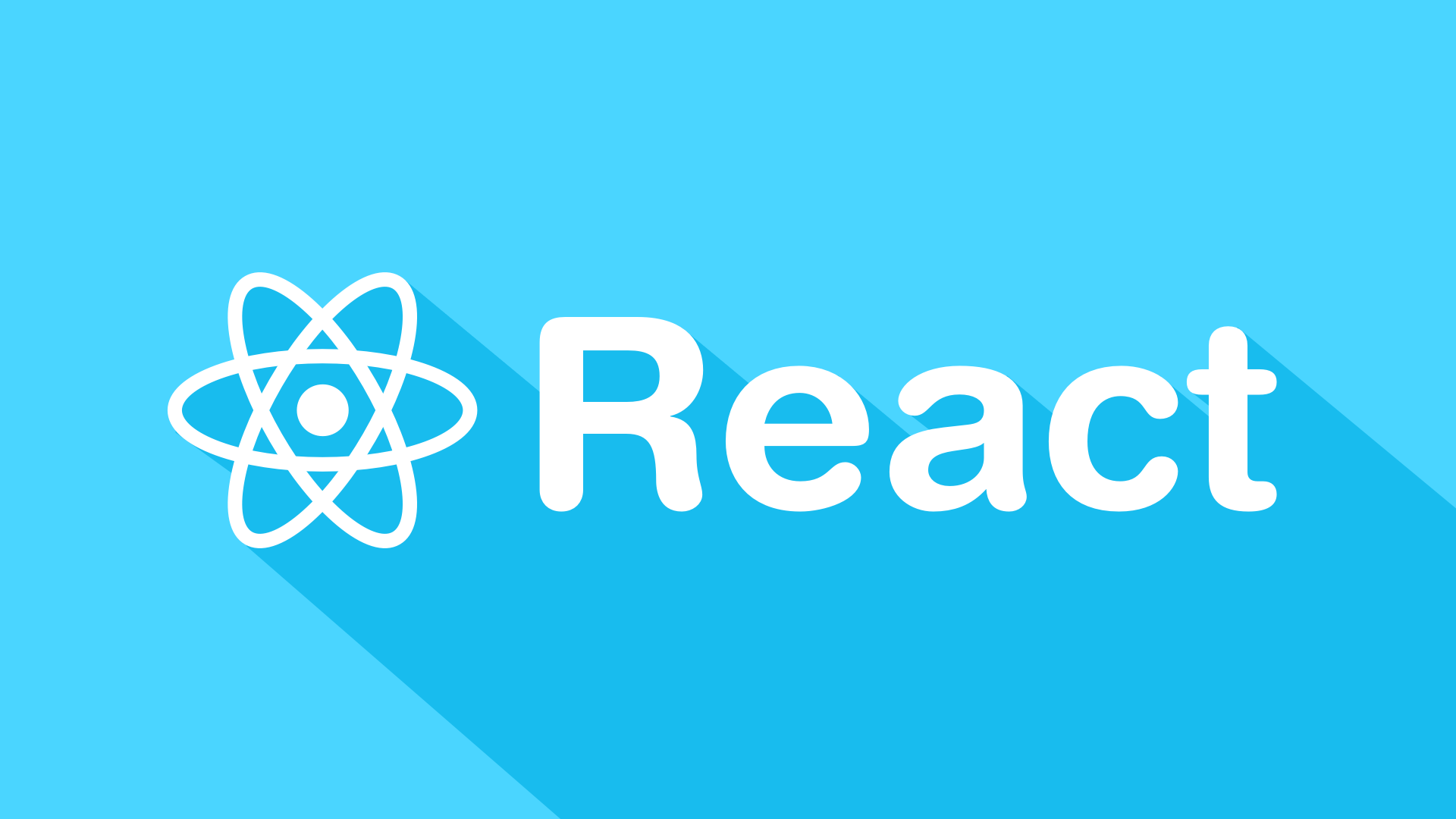
When we fetch data in our React front-end app from API, sometimes we fetch some errors. In this article, we just discuss one of them which is Uncaught TypeError: this.props.data.map is not a function. Before discuss about this error we discuss about what is .map() and how it works. The .map() is a javascript array function. It's executed with only array data and create a new array with some modification if needed. Now is the time to move eyes towards error. First we will create the same error and then we will fix it with example.
App.js
import React, { Component } from 'react';
import TodoList from "./TodoList";
class App extends Component {
constructor() {
super();
this.state = ({
todos: ""
});
}
componentDidMount() {
this.setState({
todos: [
{ id: 1, title: "Todo 1" },
{ id: 2, title: "Todo 2" },
{ id: 3, title: "Todo 3" },
{ id: 4, title: "Todo 4" },
{ id: 5, title: "Todo 5" }
]
});
}
render() {
return (
<div>
<TodoList data={ this.state.todos }/>
</div>
);
}
}
export default App;
TodoList.js
import React, { Component } from 'react';
class TodoList extends Component {
constructor(props) {
super(props);
}
render() {
return (
<ul>
{ this.props.data.map(todo => {
return (
<li key={ todo.id }>{ todo.title }</li>
);
}) }
</ul>
);
}
}
export default TodoList;
Here we have two components. One is App.js which is parent component and another one is TodoList.js which is child component of App.js component. In the App.js component, we have a todos state which initial value is a null string. We update the todos state when the component is rendered. And the todos state passed into the TodoList.js component as props. In the TodoList.js, we have been used .map() function to execute the props data.
Now if we run our project, we will see the error. If I ask you, can you tell me where is the problem? Don't worry, now I will explain to you where is the problem. We know that .map() function only executed with array data. Now we will try to find out the source of this.props.data which is executed with .map() function. this.props.data comes from parent component which is represent App.js todos state. Now here's a question. Is Todos an array? After App.js rendered Todos is an array but initially this is a null string. That's the problem. Just change the Todos initial state as an array and run the project again and see the magic.
App.js
import React, { Component } from 'react';
import TodoList from "./TodoList";
class App extends Component {
constructor() {
super();
this.state = ({
todos: [] // just update null string to array
});
}
componentDidMount() {
this.setState({
todos: [
{ id: 1, title: "Todo 1" },
{ id: 2, title: "Todo 2" },
{ id: 3, title: "Todo 3" },
{ id: 4, title: "Todo 4" },
{ id: 5, title: "Todo 5" }
]
});
}
render() {
return (
<div>
<TodoList data={ this.state.todos }/>
</div>
);
}
}
export default App;