[Solved] UnhandledPromiseRejectionWarning: Error: You must `await server.start()` before calling `server.applyMiddleware()` at ApolloServer
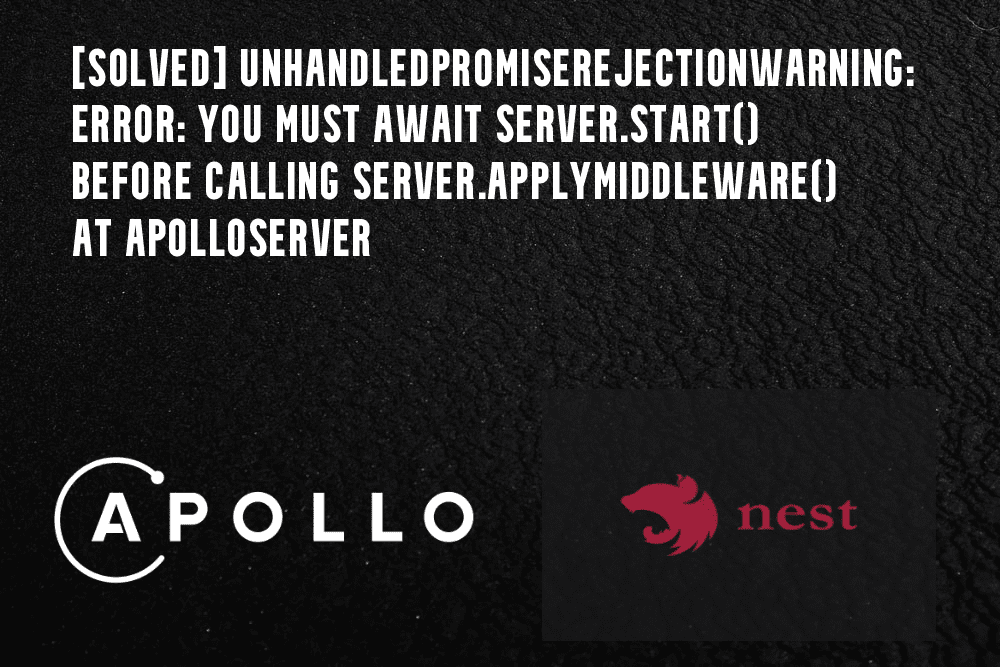
While trying to start nestJs server you may face an error that says,
UnhandledPromiseRejectionWarning: Error: You must await server.start() before calling server.applyMiddleware() at ApolloServer
In this article, we are going to show several easy solutions to solve the error.
Solution 1: downgrade to apollo-server-express@^2
First, you need to uninstall the old apollo-server-[integrations] package and replace it with the new version 2.0 of apollo-server in this case. If you're using Express, you may do so by running:
npm uninstall --save apollo-server-express npm i apollo-server@2.0.0 graphql //or use any of your required version above @2
The built-in server in Apollo Server 2.0 eliminates the need to start a server (e.g., Express, Koa, etc.).
Solution 2: Using apollo-server-express@^3.0.0
The latest version of apollo-server-express@^3.0.0 has already fixed the issue. So you can use the version and run your server without any error.
npm i apollo-server@3.1.1 //or use any of your required version above @3
Solution 3: Use await server.start() (for express)
First, you need to install any apollo version above @2. You can try v2.22.0-alpha.0 or whichever Apollo Server packages your program directly depends on. To install alpha run
npm install apollo-server-express@2.22.0-alpha.0 apollo-server-core@2.22.0-alpha.0
That should be enough If you're using the apollo-server package and calling listen().
For apollo-server-express, you have to add await server.start() between server = new ApolloServer and server.applyMiddleware. This call is optional, however, it is required to provide enhanced error handling. The whole code will be
const apolloServer = new ApolloServer({ introspection: true, schema: await buildSchema({ resolvers: [__dirname + '/resolvers/**/*.js'], validate: false }), context: ({ req, res }) => ({ req, res, redis: redisClient }), formatError }); // added this line to await server start await apolloServer.start(); apolloServer.applyMiddleware({ app, cors: false });
Thank you for reading the article. Hope the provided solutions will solve your error.