What do these three dots in React do?
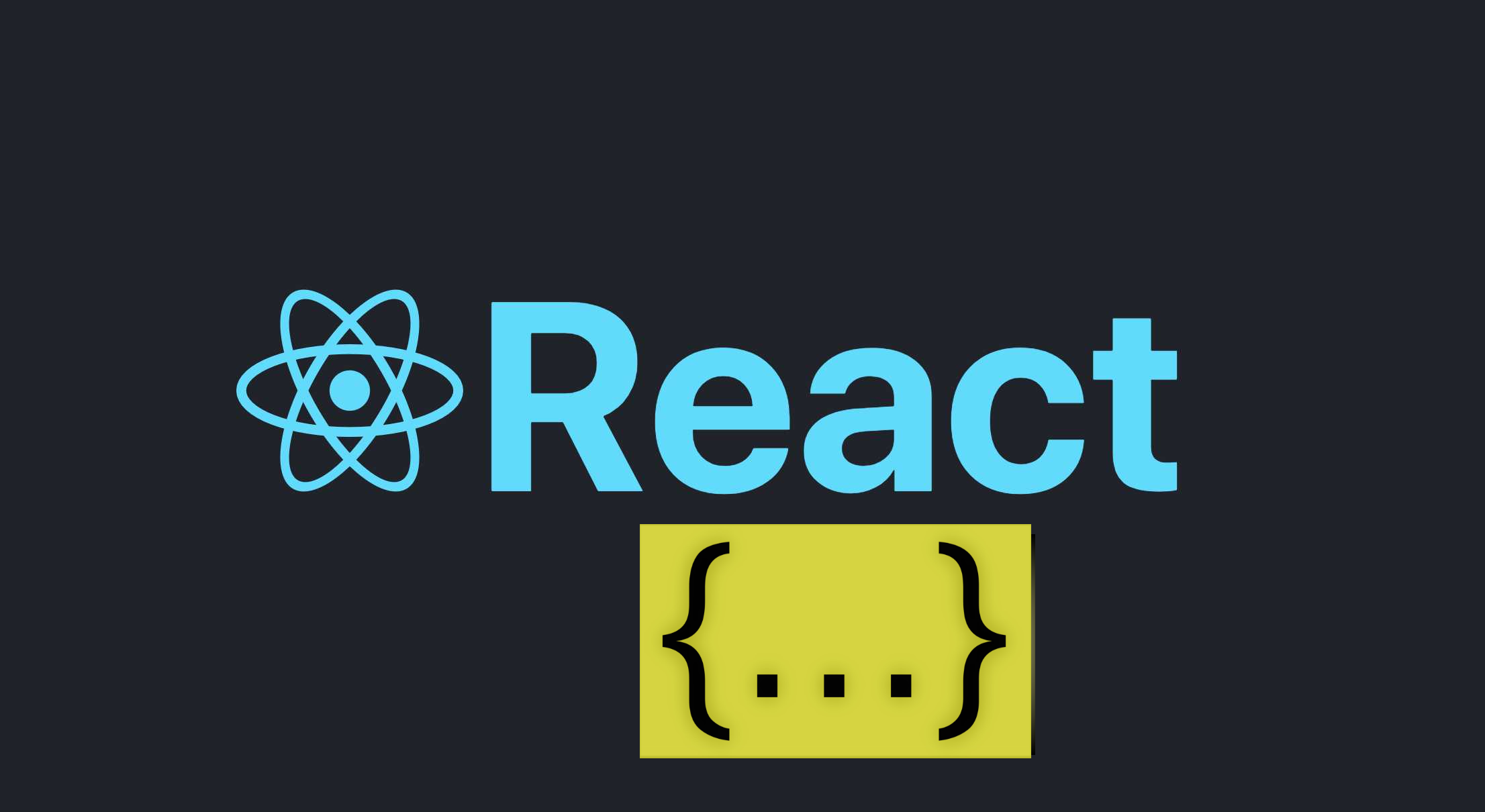
(...) operator is one of the coolest features of Javascript's ES6.
The (...) operator behaves differently in different contexts. In some contexts, it is the "spread" operator and in a different context, this could also be the "rest" operator.
Spread operators
The spread operator allows us to expand elements. Some scenarios are given below:
Adding array elements to an existing array
By using spread operator we can add elements of an array to an existing array.
const car = ["BMW", "Toyota", "Honda"]; const newCar = ["Ford", ...arr];
Here, elements of the car array will be added to newCar array.
The output of newCar array will be ["Ford", "BMW", "Toyota", "Honda"] .
It will not affect the value of the car array.
Copying an array to another array
The spread operator is used to copy an array to another array.
const letters = [‘A’, ‘B’, ‘C’]; const newLetters = [...letters ];
This copies letters array into newLetters array.
The output of newLetters will be [‘A’, ’B’, ‘C’].
It will not affect the value of the car array.
Pass elements of an array to a function as separate arguments
If we had an array that we wanted to pass as a list of arguments in a function, we would use the spread operator.
For example, we are making a sum function here which takes three parameters.
function sum(num1, num2, num3) { return num1 + num2 + num3 ; } const args = [1, 2, 3]; sum(...args);
This is similar to calling the sum function as sum(1, 2, 3).
Rest Operators
Gather arguments
Where the function’s arguments should be, either replacing the arguments completely or alongside the function’s arguments, the three dots are also called the rest operator.
With the rest operator, we can gather any number of arguments into an array and do what we want with them.
For example, we can reuse our sum function here.
function sum(...args) { let result = 0; for (let arg of args) result += arg; return result } sum(1) // returns 1 sum(1,2) // returns 3 sum(1, 2, 3, 4, 5) // returns 15
Use alongside the function’s arguments
Rest operators can also be used with function arguments.
Note: Rest parameters have to be at the last argument. This is because it collects all remaining/ excess arguments into an array.
function sum( first, ...others ) { for ( var i = 0; i < others.length; i++ ) first += others[i]; return first; } sum(1,2,3,4)
Here 1 is the value of the ‘first’ argument and 2,3,4 is the value of the ‘others’ argument.