What is the difference between JSX.Element, ReactNode and ReactElement?
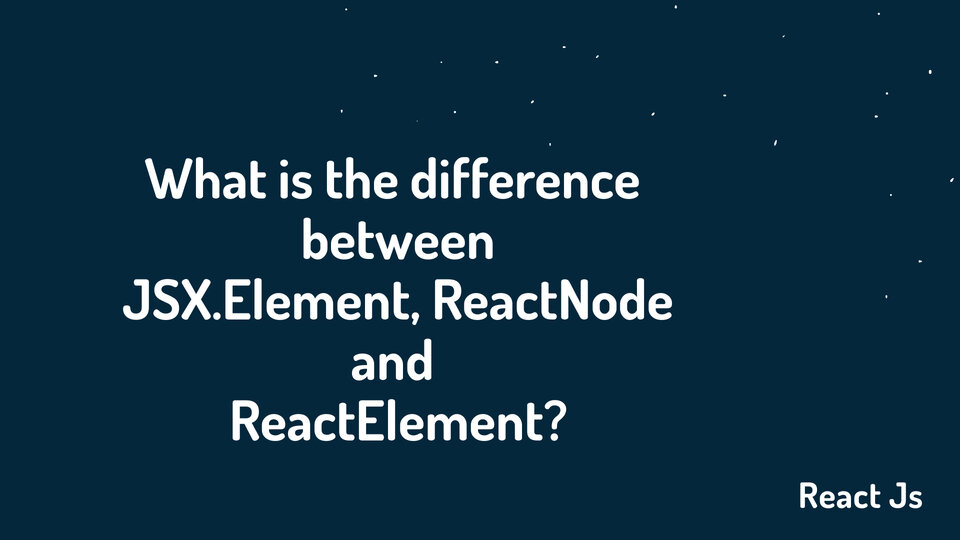
Problem:
I am working on a react project. And my project manager wants to migrate it to TypeScript. While migrating I have come across some problems. The JSX.Element does not work if a component does not render. Then, I am trying to use the ReactNode type. Some suggest that I should use ReactElement instead. So, help me understand the difference between JSX.ELement, ReactNode and ReactElement.
So in this article, we are going to learn what is the difference between JSX.Element, ReactNode and ReactElement?
Solution:
These three types usually confuse the beginner react developer. It seems like they are the same but that's not it:
1. ReactElement: This is an object which contains type, props, and key.
interface ReactElement<P = any, T extends string | JSXElementConstructor<any> = string | JSXElementConstructor<any>> {
type: T;
props: P;
key: Key | null;
}
Here, the type is defined.
2. ReactNode: This is part of ReactElement. It is a representation of virtual DOM. So it is the set of all possible return values of a component. In class components, ReactNode is used as a return type for render()
type ReactFragment = {} | Iterable<ReactNode>;
type ReactChild = ReactElement | ReactText;
type ReactText = string | number;
interface ReactPortal extends ReactElement {
key: Key | null;
children: ReactNode;
}
type ReactNode = ReactChild | ReactFragment | ReactPortal | boolean | null | undefined;
3. JSX.Element: This is also a ReactElement. JSX.Element is more of a generic type. The props and type have the type any.
declare global {
namespace JSX {
interface Element extends React.ReactElement<any, any> { }
}
}
Thank you for reading this article. If you have any more questions you can comment below.