When to use Interface and Model in TypeScript / Angular
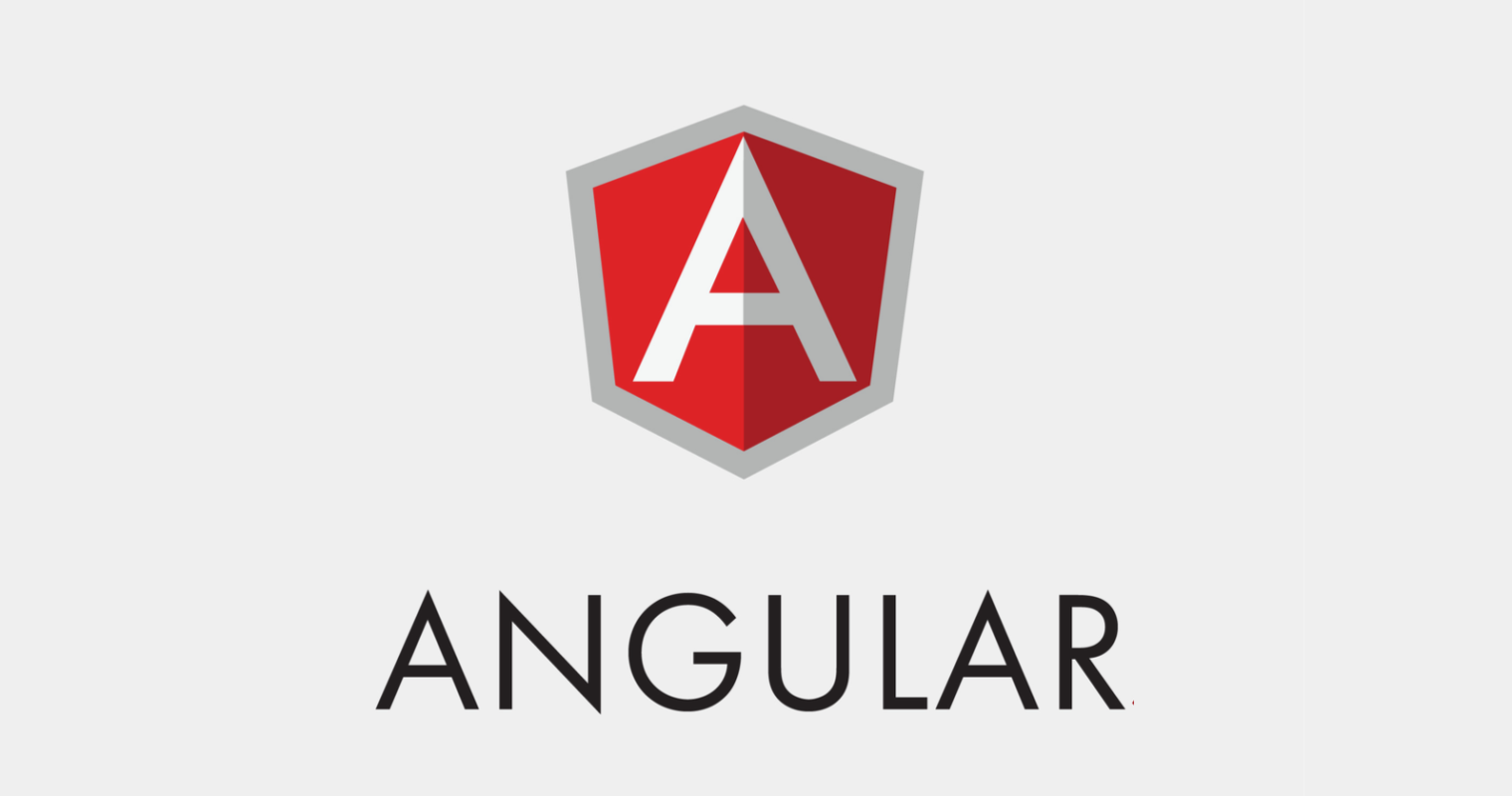
Question:
I recently watched a Tutorial on Angular 2 with TypeScript, but unsure when to use an Interface and when to use a Model for data structures.
Why Interfaces?
interface is a virtual structure that only exists within the context of TypeScript. The TypeScript compiler uses interfaces solely for type-checking purposes. Once your code is transpiled to its target language, it will be stripped from its interfaces - JavaScript isn’t typed.
interface User { id: number; username: string; } // inheritance interface UserDetails extends User { birthdate: Date; biography?: string; // use the '?' annotation to mark this property as optionnal }
Mapping server response to an interface is straight forward if you are using HttpClient from HttpClientModule if you are using latest angular version.
getUsers() :Observable<User[]> { return this.http.get<User[]>(url); // no need for '.map((res: Response) => res.json())' }when to use interfaces:
- You only need the definition for the server data without introducing additional overhead for the final output.
- You only need to transmit data without any behaviors or logic (constructor initialization, methods)
- You do not instantiate/create objects from your interface very often
- You need to define contracts/configurations for your systems (global configurations)
Why Classes?
A class defines the blueprints of an object. They express the logic, methods, and properties these objects will inherit.
class User { id: number; username: string; constructor(id :number, username: string) { this.id = id; this.username = username.replace(/^\s+|\s+$/g, ''); // trim whitespaces and new lines } } // inheritance class UserDetails extends User { birthdate: Date; biography?: string; constructor(id :number, username: string, birthdate:Date, biography? :string ) { super(id,username); this.birthdate = ...; } }when to use classes:
- You instantiate your class and change the instances state over time.
- Instances of your class will need methods to query or mutate its state
- When you want to associate behaviors with data more closely;
- You enforce constraints on the creation of your instances.
- If you only write a bunch of properties assignments in your class, you might consider using a type instead.