[Solved] Adding a HTTP header to the Angular HttpClient doesn't send the header, why?
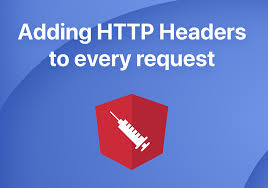
Here is my code:
import { HttpClient, HttpErrorResponse, HttpHeaders } from '@angular/common/http'; logIn(username: string, password: string) { const url = 'http://server.com/index.php'; const body = JSON.stringify({username: username, password: password}); const headers = new HttpHeaders(); headers.set('Content-Type', 'application/json; charset=utf-8'); this.http.post(url, body, {headers: headers}).subscribe( (data) => { console.log(data); }, (err: HttpErrorResponse) => { if (err.error instanceof Error) { console.log('Client-side error occured.'); } else { console.log('Server-side error occured.'); } }); }
and here the network debug: -
Request Method:POST
Status Code:200 OK
Accept:application/json, text/plain, */*
Accept-Encoding:gzip, deflate
Accept-Language:en-US,en;q=0.8
Cache-Control:no-cache
Connection:keep-alive
Content-Length:46
Content-Type:text/plain
Solution:
The instance of Httpheaders are class are unchangeable object.
When a method calls from a class it will return a new instance with new result. Headers are reset automatically. That’s why header is empty when you send a request from client side.
So, you need to follow this process –
let headers = new HttpHeaders(); headers = headers.set('Content-Type', 'application/json; charset=utf-8');
Or
const headers = new HttpHeaders({'Content-Type':'application/json; charset=utf-8'});
adding multiple headers -
let headers = new HttpHeaders(); headers = headers.set('h1', 'v1').set('h2','v2');
Or
const headers = new HttpHeaders({'h1':'v1','h2':'v2'});
From Angular latest version after V5 you can use it with –
this.http.get(url, {headers: new HttpHeaders().set('UserEmail', email ) });