How to call child method from parent in react functional component
Article
Ab Siddik
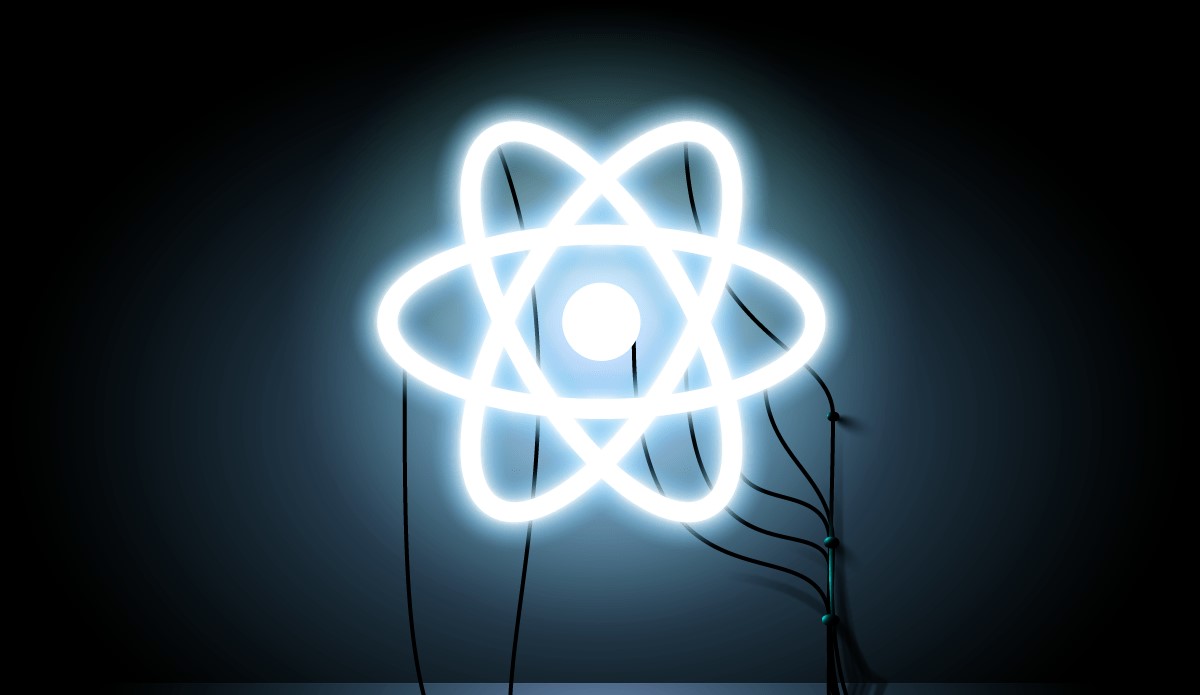
In React sometime we need to call child method from parent in react.
Parent Componentimport Child from "./Child";
export default function App() {
return (
<div className="App">
<Child />
<button>Change message</button>
</div>
);
}
Child Component
import { useState } from "react";
const Child = () => {
const [mess, setMess] = useState("Hello World!!!");
const changeMessage = (mess) => {
setMess(mess);
};
return <p>{mess}</p>;
}
export default Child;
If we want to update message, we need to call changeMessage method from child component. Now i will explain how to call child method from parent. In React functional component has lots of hooks. We use useRef hook to solve this issue.
Parent Componentimport Child from "./Child";
import { useRef } from "react";
export default function App() {
const childRef = useRef(null);
const updateMessage = () => {
childRef.current.changeMessage("Hi, Namespace IT");
};
return (
<div className="App">
<Child ref={childRef} />
<button onClick={updateMessage}>Change message</button>
</div>
);
}
Child Component
import { forwardRef, useState, useImperativeHandle } from "react";
const Child = forwardRef((props, ref) => {
const [mess, setMess] = useState("Hello World!!!");
useImperativeHandle(ref, () => ({
changeMessage(mess) {
setMess(mess);
}
}));
return <p>{mess}</p>;
});
export default Child;