How to change React-Hook-Form defaultValue with useEffect()?
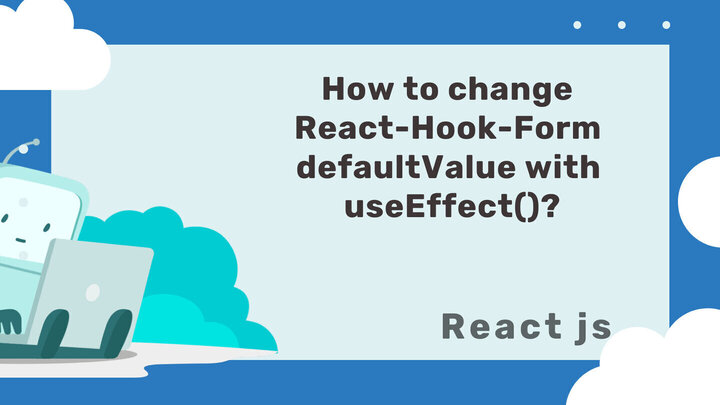
Problem:
You created a form using react-hook-form. and no you are wondering how to change React-Hook-Form defaultValue with useEffect()?
import React, {useState, useEffect, useCallback} from 'react';
import { useForm, Controller } from 'react-hook-form';
return (
<div>
<form>
<div>
<label>Name:</label>
<Controller
as={<input type='text' />}
defaultValue={data ? data.userName : ' ' }
name='name'
/>
</div>
<div>
<label>Phone:</label>
<Controller
as={<input type='text' />}
defaultValue={data ? data.age : ' ' }
name='age'
/>
</div>
</form>
</div>
);
}
export default CreateUserData;
You can do it in many ways using useEffect() , useMemo(). Here is some solution with examples:
Solution 1:
To change react-hook-form defaultValue with useEffect(). You can use setValue from useFrom. Let me give you an example:
import React, { useEffect} from 'react';
import { useForm } from 'react-hook-form'
const { setValue} = useForm({ mode: 'onBlur' });
Get setValue from useForm(); then get the data you want to change after it’s received:
useEffect(() => {
if (data) {
setValue([
{ useName: data.userName },
{ age: data.age}
]);
}
}, [data]);
This is how you can change the default values. Also if this does not work you can check the other solution below.
Solution 2:
This solution works on version 6.15.1.
import React, { useEffect} from 'react';
import { useForm } from 'react-hook-form'
const { setValue} = useForm({ mode: 'onBlur' });
useEffect(() => {
for(const [id, value] of Object.entries(data)){
setValue(id, value, {
shouldValidate: true,
shouldDirty: true
})
}
}, [data]);
const {reset} = useForm({
defaultValues: useMemo(() => {
return props.data;
}, [props])
});
after that, you have to listen to change using useEffect() hook.
useEffect(() => {
reset(props.data);
}, [props.data]);
If these solutions do not help with the problem you are facing, then please comment below. We will be very much happy to help you.