[Solved] How to fix "don't use object as a type" error in typescript?
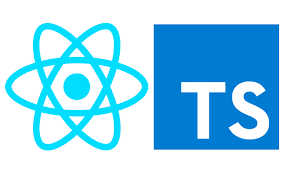
In ReactJS, typescript is a part of react app development. It makes app more reliable and easier to refactor. Sometimes we face some issues in typescript. Object type is one of them. In this article, we will try to describe about react object typescript.
We need an object type to define an Object. Imagine, we have a User object which has name, email and phone properties. So if we want to define User object, we need a User interface.
const userObject = {
name: 'Alex',
email: 'alex@example.com',
phone: 777999888
}
Now we define an Object type for this userObject.
interface User {
name: string,
email: string,
phone: number
}
Now we implement in userObject
const userObject: User = {
name: 'Alex',
email: 'alex@example.com',
phone: 777999888
}
If you want to more properties in userObject, you must be declared in User interface. Otherwise it will show an error.