Loop inside React JSX | Use "for loop" in JSX
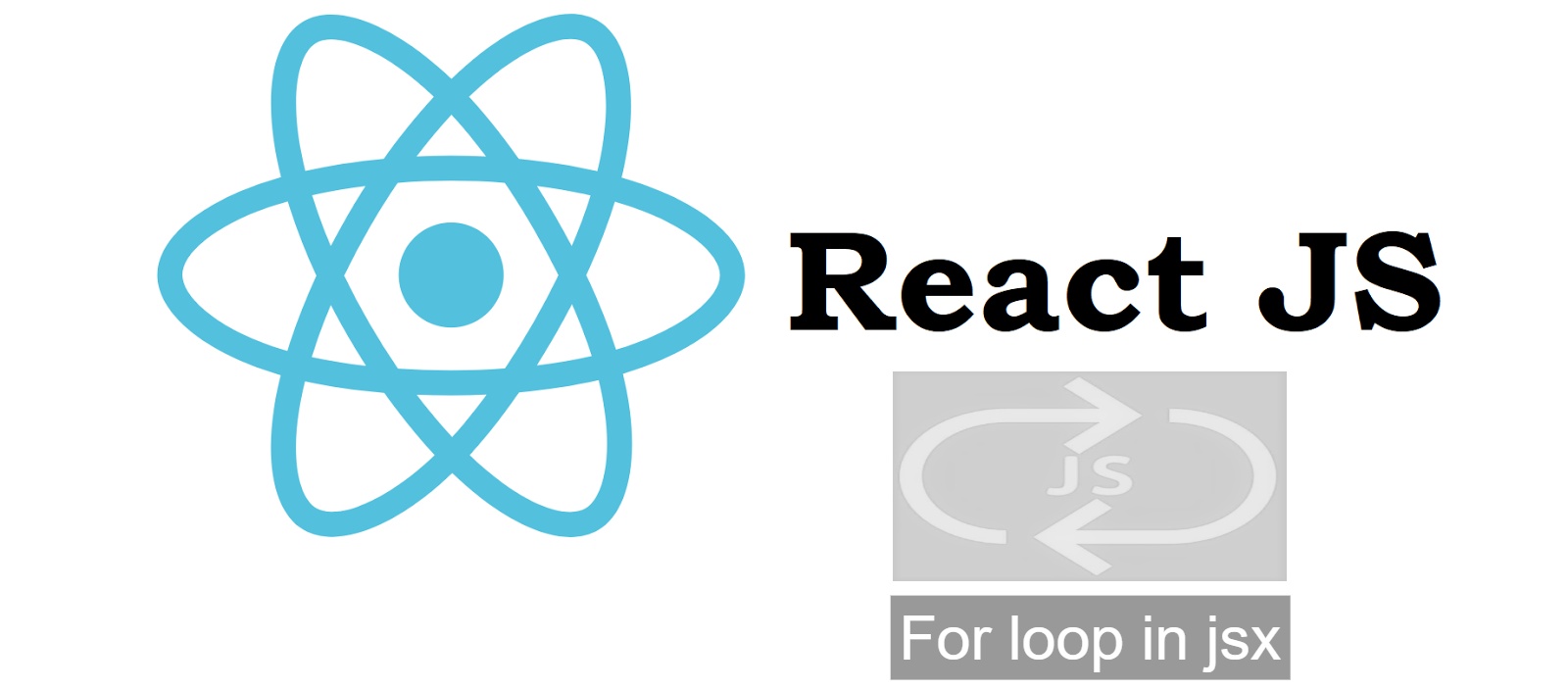
Most of the time, developers use the map function in jsx to render a list of items. Because map does exactly the same thing as what the for loop does, except that map creates a new array with the result of calling a provided function on every element in the calling array. But sometimes, a situation may occur when you need to use a for loop in your jsx. Because map method is suitable for iterating an array and for loop is suitable for iterating numbers.
A typical for loop is not a javascript expression because it does not return anything, which is why we have to push values into an array and return the array. So, we have to make a helper function for that. We will implement the loop in the function and push the component into an array. And after completing the iteration we will return the array.
function ComponentForLoop() { const rows = []; for (let i = 0; i < 5; i++) { rows.push( <LoopComponent /> ); } return <div>{rows}</div>; }
Then, we will call the helper function in our component’s return method inside the curly braces, and it will call the LoopComponent 5 times.
function App() { return ( <div className="App"> { ComponentForLoop() } </div> ); }
Finally, our whole component will look like this,
function ComponentForLoop() { const rows = []; for (let i = 0; i < 5; i++) { rows.push( <LoopComponent /> ); } return <div>{rows}</div>; } function App() { return ( <div className="App"> { ComponentForLoop() } {/* or use the helper function as a component if you need to send a props through it <ComponentForLoop /> */} </div> ); }