[Solved] React JSX: selecting “selected” on selected select option
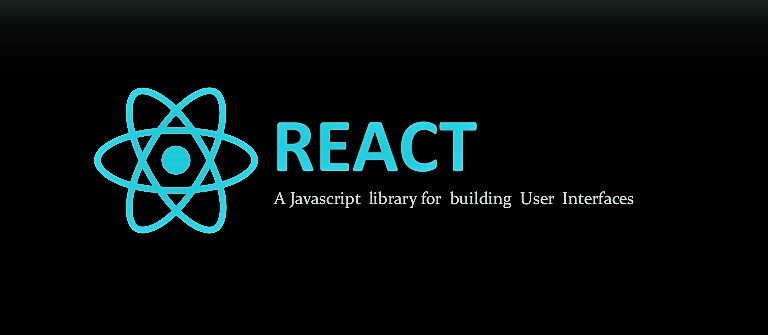
While using React Js, you may need a pre-selected option in your dropdown selection field. For this, you may want to send a selection field value from parent component to child component as props. Then in the child component, you want to use the props value and pre-select the right option in the select dropdown field.
To achieve the goal, you have to first set your desired selection field option value in a state of your parent component and send it as props in your child component.
class ParentComponent extends Component { state = { car: 'porsche' //or you can use a dynamic value fetching from your server }; render() { return ( <div> <ChildComponent car={this.state.car}/> </div> ); } }
Here, we sent a value as props from our parent component to the child component. Now, we can use the props value in our child component and set it in the state of the child component.
class ChildComponent extends React.Component { constructor(props) { super(props); this.state = {value: props.car}; this.handleChange = this.handleChange.bind(this); this.handleSubmit = this.handleSubmit.bind(this); }
Here we need two additional methods. handleChange() method is to handle the changes of your dropdown field value and handleSubmit() is to submit the form.
handleChange(event) { this.setState({value: event.target.value}); } handleSubmit(event) { alert('Your favorite car brand is: ' + this.state.value); event.preventDefault(); }
Finally, we will use your state value of child component to pre-select the selection field option in our select tag like this
<select value={this.state.value}>
So, our whole render function will be,
render() { return ( <form onSubmit={this.handleSubmit}> <label> Pick your favorite car brand: <select value={this.state.value} onChange={this.handleChange}> <option value="bmw">BMW</option> <option value="toyota">Toyota</option> <option value="jeep">Jeep</option> <option value="porsche">Porsche</option> </select> </label> <input type="submit" value="Submit" /> </form> ); }